Optional[Sho
async def add_item(
- shop: int, name: str, description: str, image: Optional[str], price: int, unit: str
+ shop: int,
+ name: str,
+ description: str,
+ image: Optional[str],
+ price: int,
+ unit: str,
+ fiat_base_multiplier: int,
) -> int:
result = await db.execute(
"""
- INSERT INTO offlineshop.items (shop, name, description, image, price, unit)
- VALUES (?, ?, ?, ?, ?, ?)
+ INSERT INTO offlineshop.items (shop, name, description, image, price, unit, fiat_base_multiplier)
+ VALUES (?, ?, ?, ?, ?, ?, ?)
""",
- (shop, name, description, image, price, unit),
+ (shop, name, description, image, price, unit, fiat_base_multiplier),
)
return result._result_proxy.lastrowid
@@ -72,6 +78,7 @@ async def update_item(
image: Optional[str],
price: int,
unit: str,
+ fiat_base_multiplier: int,
) -> int:
await db.execute(
"""
@@ -80,10 +87,11 @@ async def update_item(
description = ?,
image = ?,
price = ?,
- unit = ?
+ unit = ?,
+ fiat_base_multiplier = ?
WHERE shop = ? AND id = ?
""",
- (name, description, image, price, unit, shop, item_id),
+ (name, description, image, price, unit, fiat_base_multiplier, shop, item_id),
)
return item_id
@@ -92,12 +100,12 @@ async def get_item(id: int) -> Optional[Item]:
row = await db.fetchone(
"SELECT * FROM offlineshop.items WHERE id = ? LIMIT 1", (id,)
)
- return Item(**dict(row)) if row else None
+ return Item.from_row(row) if row else None
async def get_items(shop: int) -> List[Item]:
rows = await db.fetchall("SELECT * FROM offlineshop.items WHERE shop = ?", (shop,))
- return [Item(**dict(row)) for row in rows]
+ return [Item.from_row(row) for row in rows]
async def delete_item_from_shop(shop: int, item_id: int):
diff --git a/lnbits/extensions/offlineshop/migrations.py b/lnbits/extensions/offlineshop/migrations.py
index f7c2dfec8..84aea27e2 100644
--- a/lnbits/extensions/offlineshop/migrations.py
+++ b/lnbits/extensions/offlineshop/migrations.py
@@ -27,3 +27,13 @@ async def m001_initial(db):
);
"""
)
+
+
+async def m002_fiat_base_multiplier(db):
+ """
+ Store the multiplier for fiat prices. We store the price in cents and
+ remember to multiply by 100 when we use it to convert to Dollars.
+ """
+ await db.execute(
+ "ALTER TABLE offlineshop.items ADD COLUMN fiat_base_multiplier INTEGER DEFAULT 1;"
+ )
diff --git a/lnbits/extensions/offlineshop/models.py b/lnbits/extensions/offlineshop/models.py
index 0128fdb84..ca5c73a55 100644
--- a/lnbits/extensions/offlineshop/models.py
+++ b/lnbits/extensions/offlineshop/models.py
@@ -2,6 +2,7 @@ import base64
import hashlib
import json
from collections import OrderedDict
+from sqlite3 import Row
from typing import Dict, List, Optional
from lnurl import encode as lnurl_encode # type: ignore
@@ -87,8 +88,16 @@ class Item(BaseModel):
description: str
image: Optional[str]
enabled: bool
- price: int
+ price: float
unit: str
+ fiat_base_multiplier: int
+
+ @classmethod
+ def from_row(cls, row: Row) -> "Item":
+ data = dict(row)
+ if data["unit"] != "sat" and data["fiat_base_multiplier"]:
+ data["price"] /= data["fiat_base_multiplier"]
+ return cls(**data)
def lnurl(self, req: Request) -> str:
return lnurl_encode(req.url_for("offlineshop.lnurl_response", item_id=self.id))
diff --git a/lnbits/extensions/offlineshop/static/js/index.js b/lnbits/extensions/offlineshop/static/js/index.js
index 00e932416..c03906093 100644
--- a/lnbits/extensions/offlineshop/static/js/index.js
+++ b/lnbits/extensions/offlineshop/static/js/index.js
@@ -124,7 +124,8 @@ new Vue({
description,
image,
price,
- unit
+ unit,
+ fiat_base_multiplier: unit == 'sat' ? 1 : 100
}
try {
diff --git a/lnbits/extensions/offlineshop/views_api.py b/lnbits/extensions/offlineshop/views_api.py
index 5ced43516..1bd045dd9 100644
--- a/lnbits/extensions/offlineshop/views_api.py
+++ b/lnbits/extensions/offlineshop/views_api.py
@@ -1,6 +1,7 @@
from http import HTTPStatus
from typing import Optional
+from fastapi import Query
from fastapi.params import Depends
from lnurl.exceptions import InvalidUrl as LnurlInvalidUrl
from pydantic.main import BaseModel
@@ -34,7 +35,6 @@ async def api_shop_from_wallet(
):
shop = await get_or_create_shop_by_wallet(wallet.wallet.id)
items = await get_items(shop.id)
-
try:
return {
**shop.dict(),
@@ -51,8 +51,9 @@ class CreateItemsData(BaseModel):
name: str
description: str
image: Optional[str]
- price: int
+ price: float
unit: str
+ fiat_base_multiplier: int = Query(100, ge=1)
@offlineshop_ext.post("/api/v1/offlineshop/items")
@@ -61,9 +62,18 @@ async def api_add_or_update_item(
data: CreateItemsData, item_id=None, wallet: WalletTypeInfo = Depends(get_key_type)
):
shop = await get_or_create_shop_by_wallet(wallet.wallet.id)
+ if data.unit != "sat":
+ data.price = data.price * 100
if item_id == None:
+
await add_item(
- shop.id, data.name, data.description, data.image, data.price, data.unit
+ shop.id,
+ data.name,
+ data.description,
+ data.image,
+ data.price,
+ data.unit,
+ data.fiat_base_multiplier,
)
return HTMLResponse(status_code=HTTPStatus.CREATED)
else:
@@ -75,6 +85,7 @@ async def api_add_or_update_item(
data.image,
data.price,
data.unit,
+ data.fiat_base_multiplier,
)
diff --git a/lnbits/extensions/satspay/templates/satspay/index.html b/lnbits/extensions/satspay/templates/satspay/index.html
index 5be38cf6b..551b81b8e 100644
--- a/lnbits/extensions/satspay/templates/satspay/index.html
+++ b/lnbits/extensions/satspay/templates/satspay/index.html
@@ -225,7 +225,8 @@
- Watch-Only extension MUST be activated and have a wallet
+ Onchain Wallet (watch-only) extension MUST be activated and
+ have a wallet
diff --git a/lnbits/extensions/scrub/README.md b/lnbits/extensions/scrub/README.md
new file mode 100644
index 000000000..680c5e6db
--- /dev/null
+++ b/lnbits/extensions/scrub/README.md
@@ -0,0 +1,28 @@
+# Scrub
+
+## Automatically forward funds (Scrub) that get paid to the wallet to an LNURLpay or Lightning Address
+
+SCRUB is a small but handy extension that allows a user to take advantage of all the functionalities inside **LNbits** and upon a payment received to your LNbits wallet, automatically forward it to your desired wallet via LNURL or LNAddress!
+
+[**Wallets supporting LNURL**](https://github.com/fiatjaf/awesome-lnurl#wallets)
+
+## Usage
+
+1. Create an scrub (New Scrub link)\
+ 
+
+ - select the wallet to be _scrubbed_
+ - make a small description
+ - enter either an LNURL pay or a lightning address
+
+ Make sure your LNURL or LNaddress is correct!
+
+2. A new scrub will show on the _Scrub links_ section\
+ 
+
+ - only one scrub can be created for each wallet!
+ - You can _edit_ or _delete_ the Scrub at any time\
+ 
+
+3. On your wallet, you'll see a transaction of a payment received and another right after it as apayment sent, marked with **#scrubed**\
+ 
diff --git a/lnbits/extensions/scrub/__init__.py b/lnbits/extensions/scrub/__init__.py
new file mode 100644
index 000000000..777a7c3f9
--- /dev/null
+++ b/lnbits/extensions/scrub/__init__.py
@@ -0,0 +1,34 @@
+import asyncio
+
+from fastapi import APIRouter
+from fastapi.staticfiles import StaticFiles
+
+from lnbits.db import Database
+from lnbits.helpers import template_renderer
+from lnbits.tasks import catch_everything_and_restart
+
+db = Database("ext_scrub")
+
+scrub_static_files = [
+ {
+ "path": "/scrub/static",
+ "app": StaticFiles(directory="lnbits/extensions/scrub/static"),
+ "name": "scrub_static",
+ }
+]
+
+scrub_ext: APIRouter = APIRouter(prefix="/scrub", tags=["scrub"])
+
+
+def scrub_renderer():
+ return template_renderer(["lnbits/extensions/scrub/templates"])
+
+
+from .tasks import wait_for_paid_invoices
+from .views import * # noqa
+from .views_api import * # noqa
+
+
+def scrub_start():
+ loop = asyncio.get_event_loop()
+ loop.create_task(catch_everything_and_restart(wait_for_paid_invoices))
diff --git a/lnbits/extensions/scrub/config.json b/lnbits/extensions/scrub/config.json
new file mode 100644
index 000000000..df9e00389
--- /dev/null
+++ b/lnbits/extensions/scrub/config.json
@@ -0,0 +1,6 @@
+{
+ "name": "Scrub",
+ "short_description": "Pass payments to LNURLp/LNaddress",
+ "icon": "send",
+ "contributors": ["arcbtc", "talvasconcelos"]
+}
diff --git a/lnbits/extensions/scrub/crud.py b/lnbits/extensions/scrub/crud.py
new file mode 100644
index 000000000..1772a8c5b
--- /dev/null
+++ b/lnbits/extensions/scrub/crud.py
@@ -0,0 +1,80 @@
+from typing import List, Optional, Union
+
+from lnbits.helpers import urlsafe_short_hash
+
+from . import db
+from .models import CreateScrubLink, ScrubLink
+
+
+async def create_scrub_link(data: CreateScrubLink) -> ScrubLink:
+ scrub_id = urlsafe_short_hash()
+ await db.execute(
+ """
+ INSERT INTO scrub.scrub_links (
+ id,
+ wallet,
+ description,
+ payoraddress
+ )
+ VALUES (?, ?, ?, ?)
+ """,
+ (
+ scrub_id,
+ data.wallet,
+ data.description,
+ data.payoraddress,
+ ),
+ )
+ link = await get_scrub_link(scrub_id)
+ assert link, "Newly created link couldn't be retrieved"
+ return link
+
+
+async def get_scrub_link(link_id: str) -> Optional[ScrubLink]:
+ row = await db.fetchone("SELECT * FROM scrub.scrub_links WHERE id = ?", (link_id,))
+ return ScrubLink(**row) if row else None
+
+
+async def get_scrub_links(wallet_ids: Union[str, List[str]]) -> List[ScrubLink]:
+ if isinstance(wallet_ids, str):
+ wallet_ids = [wallet_ids]
+
+ q = ",".join(["?"] * len(wallet_ids))
+ rows = await db.fetchall(
+ f"""
+ SELECT * FROM scrub.scrub_links WHERE wallet IN ({q})
+ ORDER BY id
+ """,
+ (*wallet_ids,),
+ )
+ return [ScrubLink(**row) for row in rows]
+
+
+async def update_scrub_link(link_id: int, **kwargs) -> Optional[ScrubLink]:
+ q = ", ".join([f"{field[0]} = ?" for field in kwargs.items()])
+ await db.execute(
+ f"UPDATE scrub.scrub_links SET {q} WHERE id = ?",
+ (*kwargs.values(), link_id),
+ )
+ row = await db.fetchone("SELECT * FROM scrub.scrub_links WHERE id = ?", (link_id,))
+ return ScrubLink(**row) if row else None
+
+
+async def delete_scrub_link(link_id: int) -> None:
+ await db.execute("DELETE FROM scrub.scrub_links WHERE id = ?", (link_id,))
+
+
+async def get_scrub_by_wallet(wallet_id) -> Optional[ScrubLink]:
+ row = await db.fetchone(
+ "SELECT * from scrub.scrub_links WHERE wallet = ?",
+ (wallet_id,),
+ )
+ return ScrubLink(**row) if row else None
+
+
+async def unique_scrubed_wallet(wallet_id):
+ (row,) = await db.fetchone(
+ "SELECT COUNT(wallet) FROM scrub.scrub_links WHERE wallet = ?",
+ (wallet_id,),
+ )
+ return row
diff --git a/lnbits/extensions/scrub/migrations.py b/lnbits/extensions/scrub/migrations.py
new file mode 100644
index 000000000..f8f2ba43c
--- /dev/null
+++ b/lnbits/extensions/scrub/migrations.py
@@ -0,0 +1,14 @@
+async def m001_initial(db):
+ """
+ Initial scrub table.
+ """
+ await db.execute(
+ f"""
+ CREATE TABLE scrub.scrub_links (
+ id TEXT PRIMARY KEY,
+ wallet TEXT NOT NULL,
+ description TEXT NOT NULL,
+ payoraddress TEXT NOT NULL
+ );
+ """
+ )
diff --git a/lnbits/extensions/scrub/models.py b/lnbits/extensions/scrub/models.py
new file mode 100644
index 000000000..db05e4f17
--- /dev/null
+++ b/lnbits/extensions/scrub/models.py
@@ -0,0 +1,28 @@
+from sqlite3 import Row
+
+from pydantic import BaseModel
+from starlette.requests import Request
+
+from lnbits.lnurl import encode as lnurl_encode # type: ignore
+
+
+class CreateScrubLink(BaseModel):
+ wallet: str
+ description: str
+ payoraddress: str
+
+
+class ScrubLink(BaseModel):
+ id: str
+ wallet: str
+ description: str
+ payoraddress: str
+
+ @classmethod
+ def from_row(cls, row: Row) -> "ScrubLink":
+ data = dict(row)
+ return cls(**data)
+
+ def lnurl(self, req: Request) -> str:
+ url = req.url_for("scrub.api_lnurl_response", link_id=self.id)
+ return lnurl_encode(url)
diff --git a/lnbits/extensions/scrub/static/js/index.js b/lnbits/extensions/scrub/static/js/index.js
new file mode 100644
index 000000000..439907921
--- /dev/null
+++ b/lnbits/extensions/scrub/static/js/index.js
@@ -0,0 +1,143 @@
+/* globals Quasar, Vue, _, VueQrcode, windowMixin, LNbits, LOCALE */
+
+Vue.component(VueQrcode.name, VueQrcode)
+
+var locationPath = [
+ window.location.protocol,
+ '//',
+ window.location.host,
+ window.location.pathname
+].join('')
+
+var mapScrubLink = obj => {
+ obj._data = _.clone(obj)
+ obj.date = Quasar.utils.date.formatDate(
+ new Date(obj.time * 1000),
+ 'YYYY-MM-DD HH:mm'
+ )
+ obj.amount = new Intl.NumberFormat(LOCALE).format(obj.amount)
+ obj.print_url = [locationPath, 'print/', obj.id].join('')
+ obj.pay_url = [locationPath, obj.id].join('')
+ return obj
+}
+
+new Vue({
+ el: '#vue',
+ mixins: [windowMixin],
+ data() {
+ return {
+ checker: null,
+ payLinks: [],
+ payLinksTable: {
+ pagination: {
+ rowsPerPage: 10
+ }
+ },
+ formDialog: {
+ show: false,
+ data: {}
+ },
+ qrCodeDialog: {
+ show: false,
+ data: null
+ }
+ }
+ },
+ methods: {
+ getScrubLinks() {
+ LNbits.api
+ .request(
+ 'GET',
+ '/scrub/api/v1/links?all_wallets=true',
+ this.g.user.wallets[0].inkey
+ )
+ .then(response => {
+ this.payLinks = response.data.map(mapScrubLink)
+ })
+ .catch(err => {
+ clearInterval(this.checker)
+ LNbits.utils.notifyApiError(err)
+ })
+ },
+ closeFormDialog() {
+ this.resetFormData()
+ },
+ openUpdateDialog(linkId) {
+ const link = _.findWhere(this.payLinks, {id: linkId})
+
+ this.formDialog.data = _.clone(link._data)
+ this.formDialog.show = true
+ },
+ sendFormData() {
+ const wallet = _.findWhere(this.g.user.wallets, {
+ id: this.formDialog.data.wallet
+ })
+ let data = Object.freeze(this.formDialog.data)
+ console.log(wallet, data)
+
+ if (data.id) {
+ this.updateScrubLink(wallet, data)
+ } else {
+ this.createScrubLink(wallet, data)
+ }
+ },
+ resetFormData() {
+ this.formDialog = {
+ show: false,
+ data: {}
+ }
+ },
+ updateScrubLink(wallet, data) {
+ LNbits.api
+ .request('PUT', '/scrub/api/v1/links/' + data.id, wallet.adminkey, data)
+ .then(response => {
+ this.payLinks = _.reject(this.payLinks, obj => obj.id === data.id)
+ this.payLinks.push(mapScrubLink(response.data))
+ this.formDialog.show = false
+ this.resetFormData()
+ })
+ .catch(err => {
+ LNbits.utils.notifyApiError(err)
+ })
+ },
+ createScrubLink(wallet, data) {
+ LNbits.api
+ .request('POST', '/scrub/api/v1/links', wallet.adminkey, data)
+ .then(response => {
+ console.log('RES', response)
+ this.getScrubLinks()
+ this.formDialog.show = false
+ this.resetFormData()
+ })
+ .catch(err => {
+ LNbits.utils.notifyApiError(err)
+ })
+ },
+ deleteScrubLink(linkId) {
+ var link = _.findWhere(this.payLinks, {id: linkId})
+
+ LNbits.utils
+ .confirmDialog('Are you sure you want to delete this pay link?')
+ .onOk(() => {
+ LNbits.api
+ .request(
+ 'DELETE',
+ '/scrub/api/v1/links/' + linkId,
+ _.findWhere(this.g.user.wallets, {id: link.wallet}).adminkey
+ )
+ .then(response => {
+ this.payLinks = _.reject(this.payLinks, obj => obj.id === linkId)
+ })
+ .catch(err => {
+ LNbits.utils.notifyApiError(err)
+ })
+ })
+ }
+ },
+ created() {
+ if (this.g.user.wallets.length) {
+ var getScrubLinks = this.getScrubLinks
+ getScrubLinks()
+ }
+ }
+})
diff --git a/lnbits/extensions/scrub/tasks.py b/lnbits/extensions/scrub/tasks.py
new file mode 100644
index 000000000..87e1364b7
--- /dev/null
+++ b/lnbits/extensions/scrub/tasks.py
@@ -0,0 +1,85 @@
+import asyncio
+import json
+from http import HTTPStatus
+from urllib.parse import urlparse
+
+import httpx
+from fastapi import HTTPException
+
+from lnbits import bolt11
+from lnbits.core.models import Payment
+from lnbits.core.services import pay_invoice
+from lnbits.tasks import register_invoice_listener
+
+from .crud import get_scrub_by_wallet
+
+
+async def wait_for_paid_invoices():
+ invoice_queue = asyncio.Queue()
+ register_invoice_listener(invoice_queue)
+
+ while True:
+ payment = await invoice_queue.get()
+ await on_invoice_paid(payment)
+
+
+async def on_invoice_paid(payment: Payment) -> None:
+ # (avoid loops)
+ if "scrubed" == payment.extra.get("tag"):
+ # already scrubbed
+ return
+
+ scrub_link = await get_scrub_by_wallet(payment.wallet_id)
+
+ if not scrub_link:
+ return
+
+ from lnbits.core.views.api import api_lnurlscan
+
+ # DECODE LNURLP OR LNADDRESS
+ data = await api_lnurlscan(scrub_link.payoraddress)
+
+ # I REALLY HATE THIS DUPLICATION OF CODE!! CORE/VIEWS/API.PY, LINE 267
+ domain = urlparse(data["callback"]).netloc
+
+ async with httpx.AsyncClient() as client:
+ try:
+ r = await client.get(
+ data["callback"],
+ params={"amount": payment.amount},
+ timeout=40,
+ )
+ if r.is_error:
+ raise httpx.ConnectError
+ except (httpx.ConnectError, httpx.RequestError):
+ raise HTTPException(
+ status_code=HTTPStatus.BAD_REQUEST,
+ detail=f"Failed to connect to {domain}.",
+ )
+
+ params = json.loads(r.text)
+ if params.get("status") == "ERROR":
+ raise HTTPException(
+ status_code=HTTPStatus.BAD_REQUEST,
+ detail=f"{domain} said: '{params.get('reason', '')}'",
+ )
+
+ invoice = bolt11.decode(params["pr"])
+ if invoice.amount_msat != payment.amount:
+ raise HTTPException(
+ status_code=HTTPStatus.BAD_REQUEST,
+ detail=f"{domain} returned an invalid invoice. Expected {payment.amount} msat, got {invoice.amount_msat}.",
+ )
+
+ payment_hash = await pay_invoice(
+ wallet_id=payment.wallet_id,
+ payment_request=params["pr"],
+ description=data["description"],
+ extra={"tag": "scrubed"},
+ )
+
+ return {
+ "payment_hash": payment_hash,
+ # maintain backwards compatibility with API clients:
+ "checking_id": payment_hash,
+ }
diff --git a/lnbits/extensions/scrub/templates/scrub/_api_docs.html b/lnbits/extensions/scrub/templates/scrub/_api_docs.html
new file mode 100644
index 000000000..ae3f44d88
--- /dev/null
+++ b/lnbits/extensions/scrub/templates/scrub/_api_docs.html
@@ -0,0 +1,136 @@
+
+
+
+
+ GET /scrub/api/v1/links
+ Headers
+ {"X-Api-Key": <invoice_key>}
+ Body (application/json)
+
+ Returns 200 OK (application/json)
+
+ [<pay_link_object>, ...]
+ Curl example
+ curl -X GET {{ request.base_url }}scrub/api/v1/links?all_wallets=true
+ -H "X-Api-Key: {{ user.wallets[0].inkey }}"
+
+
+
+
+
+
+
+ GET
+ /scrub/api/v1/links/<scrub_id>
+ Headers
+ {"X-Api-Key": <invoice_key>}
+ Body (application/json)
+
+ Returns 200 OK (application/json)
+
+ {"id": <string>, "wallet": <string>, "description":
+ <string>, "payoraddress": <string>}
+ Curl example
+ curl -X GET {{ request.base_url }}scrub/api/v1/links/<pay_id>
+ -H "X-Api-Key: {{ user.wallets[0].inkey }}"
+
+
+
+
+
+
+
+ POST /scrub/api/v1/links
+ Headers
+ {"X-Api-Key": <admin_key>}
+ Body (application/json)
+ {"wallet": <string>, "description": <string>,
+ "payoraddress": <string>}
+
+ Returns 201 CREATED (application/json)
+
+ {"id": <string>, "wallet": <string>, "description":
+ <string>, "payoraddress": <string>}
+ Curl example
+ curl -X POST {{ request.base_url }}scrub/api/v1/links -d '{"wallet":
+ <string>, "description": <string>, "payoraddress":
+ <string>}' -H "Content-type: application/json" -H "X-Api-Key: {{
+ user.wallets[0].adminkey }}"
+
+
+
+
+
+
+
+ PUT
+ /scrub/api/v1/links/<pay_id>
+ Headers
+ {"X-Api-Key": <admin_key>}
+ Body (application/json)
+ {"wallet": <string>, "description": <string>,
+ "payoraddress": <string>}
+
+ Returns 200 OK (application/json)
+
+ {"id": <string>, "wallet": <string>, "description":
+ <string>, "payoraddress": <string>}
+ Curl example
+ curl -X PUT {{ request.base_url }}scrub/api/v1/links/<pay_id>
+ -d '{"wallet": <string>, "description": <string>,
+ "payoraddress": <string>}' -H "Content-type: application/json"
+ -H "X-Api-Key: {{ user.wallets[0].adminkey }}"
+
+
+
+
+
+
+
+ DELETE
+ /scrub/api/v1/links/<pay_id>
+ Headers
+ {"X-Api-Key": <admin_key>}
+ Returns 204 NO CONTENT
+
+ Curl example
+ curl -X DELETE {{ request.base_url
+ }}scrub/api/v1/links/<pay_id> -H "X-Api-Key: {{
+ user.wallets[0].adminkey }}"
+
+
+
+
+
diff --git a/lnbits/extensions/scrub/templates/scrub/_lnurl.html b/lnbits/extensions/scrub/templates/scrub/_lnurl.html
new file mode 100644
index 000000000..da46d9c47
--- /dev/null
+++ b/lnbits/extensions/scrub/templates/scrub/_lnurl.html
@@ -0,0 +1,28 @@
+
+
+
+
+ WARNING: LNURL must be used over https or TOR
+ LNURL is a range of lightning-network standards that allow us to use
+ lightning-network differently. An LNURL-pay is a link that wallets use
+ to fetch an invoice from a server on-demand. The link or QR code is
+ fixed, but each time it is read by a compatible wallet a new QR code is
+ issued by the service. It can be used to activate machines without them
+ having to maintain an electronic screen to generate and show invoices
+ locally, or to sell any predefined good or service automatically.
+
+
+ Exploring LNURL and finding use cases, is really helping inform
+ lightning protocol development, rather than the protocol dictating how
+ lightning-network should be engaged with.
+
+ Check
+ Awesome LNURL
+ for further information.
+
+
+
diff --git a/lnbits/extensions/scrub/templates/scrub/index.html b/lnbits/extensions/scrub/templates/scrub/index.html
new file mode 100644
index 000000000..c063c858e
--- /dev/null
+++ b/lnbits/extensions/scrub/templates/scrub/index.html
@@ -0,0 +1,140 @@
+{% extends "base.html" %} {% from "macros.jinja" import window_vars with context
+%} {% block page %}
+
+
+
+
+ New scrub link
+
+
+
+
+
+
+
+ Scrub links
+
+
+
+ {% raw %}
+
+
+ Wallet
+ Description
+ LNURLPay/Address
+
+
+
+
+
+ {{ props.row.wallet }}
+ {{ props.row.description }}
+ {{ props.row.payoraddress }}
+
+
+
+
+
+
+ {% endraw %}
+
+
+
+
+
+
+
+
+ {{SITE_TITLE}} Scrub extension
+
+
+
+
+ {% include "scrub/_api_docs.html" %}
+
+ {% include "scrub/_lnurl.html" %}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Update pay link
+ Create pay link
+ Cancel
+
+
+
+
+
+{% endblock %} {% block scripts %} {{ window_vars(user) }}
+
+{% endblock %}
diff --git a/lnbits/extensions/scrub/views.py b/lnbits/extensions/scrub/views.py
new file mode 100644
index 000000000..73c7ffd9d
--- /dev/null
+++ b/lnbits/extensions/scrub/views.py
@@ -0,0 +1,18 @@
+from fastapi import Request
+from fastapi.params import Depends
+from fastapi.templating import Jinja2Templates
+from starlette.responses import HTMLResponse
+
+from lnbits.core.models import User
+from lnbits.decorators import check_user_exists
+
+from . import scrub_ext, scrub_renderer
+
+templates = Jinja2Templates(directory="templates")
+
+
+@scrub_ext.get("/", response_class=HTMLResponse)
+async def index(request: Request, user: User = Depends(check_user_exists)):
+ return scrub_renderer().TemplateResponse(
+ "scrub/index.html", {"request": request, "user": user.dict()}
+ )
diff --git a/lnbits/extensions/scrub/views_api.py b/lnbits/extensions/scrub/views_api.py
new file mode 100644
index 000000000..3714a3043
--- /dev/null
+++ b/lnbits/extensions/scrub/views_api.py
@@ -0,0 +1,112 @@
+from http import HTTPStatus
+
+from fastapi import Request
+from fastapi.param_functions import Query
+from fastapi.params import Depends
+from lnurl.exceptions import InvalidUrl as LnurlInvalidUrl # type: ignore
+from starlette.exceptions import HTTPException
+
+from lnbits.core.crud import get_user
+from lnbits.decorators import WalletTypeInfo, get_key_type, require_admin_key
+
+from . import scrub_ext
+from .crud import (
+ create_scrub_link,
+ delete_scrub_link,
+ get_scrub_link,
+ get_scrub_links,
+ unique_scrubed_wallet,
+ update_scrub_link,
+)
+from .models import CreateScrubLink
+
+
+@scrub_ext.get("/api/v1/links", status_code=HTTPStatus.OK)
+async def api_links(
+ req: Request,
+ wallet: WalletTypeInfo = Depends(get_key_type),
+ all_wallets: bool = Query(False),
+):
+ wallet_ids = [wallet.wallet.id]
+
+ if all_wallets:
+ wallet_ids = (await get_user(wallet.wallet.user)).wallet_ids
+
+ try:
+ return [link.dict() for link in await get_scrub_links(wallet_ids)]
+
+ except:
+ raise HTTPException(
+ status_code=HTTPStatus.NOT_FOUND,
+ detail="No SCRUB links made yet",
+ )
+
+
+@scrub_ext.get("/api/v1/links/{link_id}", status_code=HTTPStatus.OK)
+async def api_link_retrieve(
+ r: Request, link_id, wallet: WalletTypeInfo = Depends(get_key_type)
+):
+ link = await get_scrub_link(link_id)
+
+ if not link:
+ raise HTTPException(
+ detail="Scrub link does not exist.", status_code=HTTPStatus.NOT_FOUND
+ )
+
+ if link.wallet != wallet.wallet.id:
+ raise HTTPException(
+ detail="Not your pay link.", status_code=HTTPStatus.FORBIDDEN
+ )
+
+ return link
+
+
+@scrub_ext.post("/api/v1/links", status_code=HTTPStatus.CREATED)
+@scrub_ext.put("/api/v1/links/{link_id}", status_code=HTTPStatus.OK)
+async def api_scrub_create_or_update(
+ data: CreateScrubLink,
+ link_id=None,
+ wallet: WalletTypeInfo = Depends(require_admin_key),
+):
+ if link_id:
+ link = await get_scrub_link(link_id)
+
+ if not link:
+ raise HTTPException(
+ detail="Scrub link does not exist.", status_code=HTTPStatus.NOT_FOUND
+ )
+
+ if link.wallet != wallet.wallet.id:
+ raise HTTPException(
+ detail="Not your pay link.", status_code=HTTPStatus.FORBIDDEN
+ )
+
+ link = await update_scrub_link(**data.dict(), link_id=link_id)
+ else:
+ wallet_has_scrub = await unique_scrubed_wallet(wallet_id=data.wallet)
+ if wallet_has_scrub > 0:
+ raise HTTPException(
+ detail="Wallet is already being Scrubbed",
+ status_code=HTTPStatus.FORBIDDEN,
+ )
+ link = await create_scrub_link(data=data)
+
+ return link
+
+
+@scrub_ext.delete("/api/v1/links/{link_id}")
+async def api_link_delete(link_id, wallet: WalletTypeInfo = Depends(require_admin_key)):
+ link = await get_scrub_link(link_id)
+
+ if not link:
+ raise HTTPException(
+ detail="Scrub link does not exist.", status_code=HTTPStatus.NOT_FOUND
+ )
+
+ if link.wallet != wallet.wallet.id:
+ raise HTTPException(
+ detail="Not your pay link.", status_code=HTTPStatus.FORBIDDEN
+ )
+
+ await delete_scrub_link(link_id)
+ raise HTTPException(status_code=HTTPStatus.NO_CONTENT)
diff --git a/lnbits/extensions/streamalerts/README.md b/lnbits/extensions/streamalerts/README.md
index 726ffe767..e19ff277a 100644
--- a/lnbits/extensions/streamalerts/README.md
+++ b/lnbits/extensions/streamalerts/README.md
@@ -18,7 +18,7 @@ In the "Whitelist Users" field, input the username of a Twitch account you contr
For now, simply set the "Redirect URI" to `http://localhost`, you will change this soon.
Then, hit create:
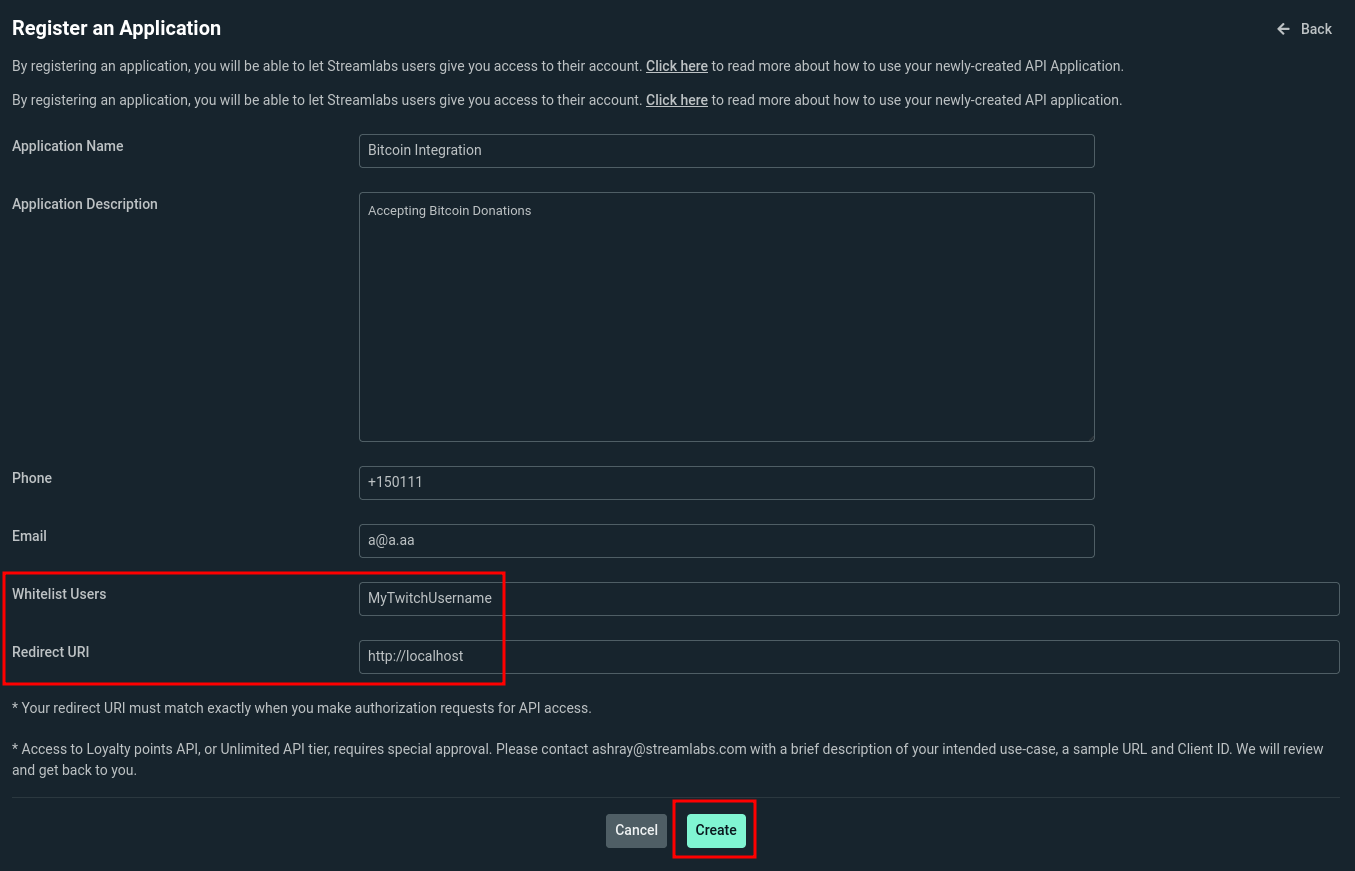
-1. In LNbits, enable the Stream Alerts extension and optionally the SatsPayServer (to observe donations directly) and Watch Only (to accept on-chain donations) extenions:
+1. In LNbits, enable the Stream Alerts extension and optionally the SatsPayServer (to observe donations directly) and Onchain Wallet (watch-only) (to accept on-chain donations) extenions:
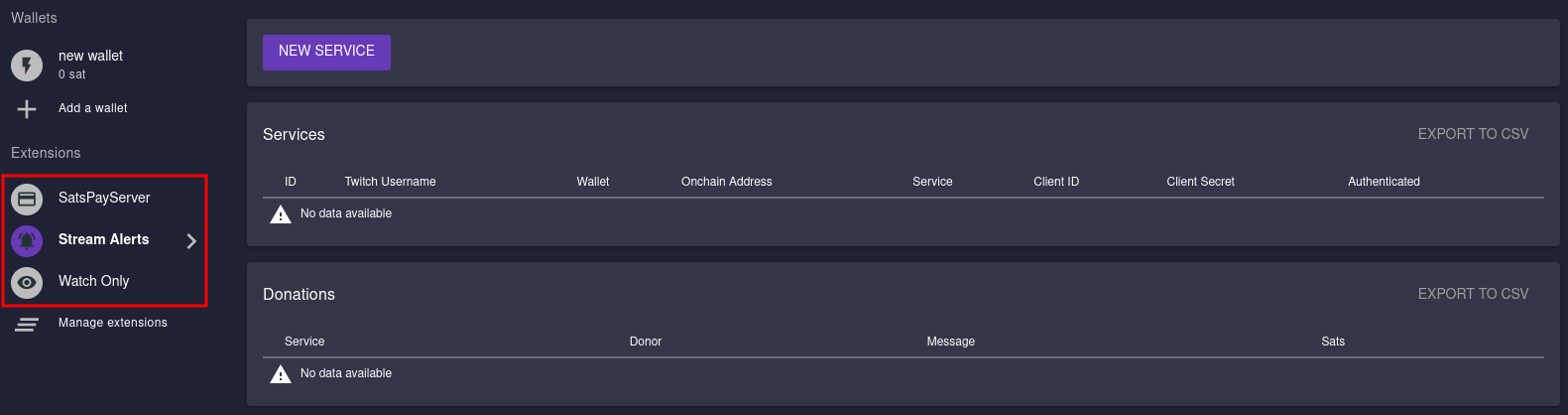
1. Create a "NEW SERVICE" using the button. Fill in all the information (you get your Client ID and Secret from the Streamlabs App page):

diff --git a/lnbits/extensions/streamalerts/templates/streamalerts/index.html b/lnbits/extensions/streamalerts/templates/streamalerts/index.html
index 46d1bb313..e86bc8b73 100644
--- a/lnbits/extensions/streamalerts/templates/streamalerts/index.html
+++ b/lnbits/extensions/streamalerts/templates/streamalerts/index.html
@@ -168,7 +168,8 @@
- Watch-Only extension MUST be activated and have a wallet
+ Onchain Wallet (watch-only) extension MUST be activated and
+ have a wallet
diff --git a/lnbits/extensions/watchonly/README.md b/lnbits/extensions/watchonly/README.md
index d93f7162d..be7bf351b 100644
--- a/lnbits/extensions/watchonly/README.md
+++ b/lnbits/extensions/watchonly/README.md
@@ -1,19 +1,85 @@
-# Watch Only wallet
+# Onchain Wallet (watch-only)
## Monitor an onchain wallet and generate addresses for onchain payments
Monitor an extended public key and generate deterministic fresh public keys with this simple watch only wallet. Invoice payments can also be generated, both through a publically shareable page and API.
-1. Start by clicking "NEW WALLET"\
- 
-2. Fill the requested fields:
- - give the wallet a name
- - paste an Extended Public Key (xpub, ypub, zpub)
- - click "CREATE WATCH-ONLY WALLET"\
- 
-3. You can then access your onchain addresses\
- 
-4. You can then generate bitcoin onchain adresses from LNbits\
- 
-
You can now use this wallet on the LNBits [SatsPayServer](https://github.com/lnbits/lnbits/blob/master/lnbits/extensions/satspay/README.md) extension
+
+### Wallet Account
+ - a user can add one or more `xPubs` or `descriptors`
+ - the `xPub` fingerprint must be unique per user
+ - such and entry is called an `Wallet Account`
+ - the addresses in a `Wallet Account` are split into `Receive Addresses` and `Change Address`
+ - the user interacts directly only with the `Receive Addresses` (by sharing them)
+ - see [BIP44](https://github.com/bitcoin/bips/blob/master/bip-0044.mediawiki#account-discovery) for more details
+ - same `xPub` will always generate the same addresses (deterministic)
+ - when a `Wallet Account` is created, there are generated `20 Receive Addresses` and `5 Change Address`
+ - the limits can be change from the `Config` page (see `screenshot 1`)
+ - regular wallets only scan up to `20` empty receive addresses. If the user generates addresses beyond this limit a warning is shown (see `screenshot 4`)
+
+### Scan Blockchain
+ - when the user clicks `Scan Blockchain`, the wallet will loop over the all addresses (for each account)
+ - if funds are found, then the list is extended
+ - will scan addresses for all wallet accounts
+ - the search is done on the client-side (using the `mempool.space` API). `mempool.space` has a limit on the number of req/sec, therefore it is expected for the scanning to start fast, but slow down as more HTTP requests have to be retried
+ - addresses can also be rescanned individually form the `Address Details` section (`Addresses` tab) of each address
+
+### New Receive Address
+ - the `New Receive Address` button show the user the NEXT un-used address
+ - un-used means funds have not already been sent to that address AND the address has not already been shared
+ - internally there is a counter that keeps track of the last shared address
+ - it is possible to add a `Note` to each address in order to remember when/with whom it was shared
+ - mind the gap (`screenshot 4`)
+
+### Addresses Tab
+- the `Addresses` tab contains a list with the addresses for all the `Wallet Accounts`
+ - only one entry per address will be shown (even if there are multiple UTXOs at that address)
+ - several filter criteria can be applied
+ - unconfirmed funds are also taken into account
+ - `Address Details` can be viewed by clicking the `Expand` button
+
+### History Tap
+ - shows the chronological order of transactions
+ - it shows unconfirmed transactions at the top
+ - it can be exported as CSV file
+
+### Coins Tab
+ - shows the UTXOs for all wallets
+ - there can be multiple UTXOs for the same address
+
+### Make Payment
+ - create a new `Partially Signed Bitcoin Transaction`
+ - multiple `Send Addresses` can be added
+ - the `Max` button next to an address is for sending the remaining funds to this address (no change)
+ - the user can select the inputs (UTXOs) manually, or it can use of the basic selection algorithms
+ - amounts have to be provided for the `Send Addresses` beforehand (so the algorithm knows the amount to be selected)
+ - `Show Advanced` allows to (see `screenshot 2`):
+ - select from which account the change address will be selected (defaults to the first one)
+ - select the `Fee Rate`
+ - it defaults to the `Medium` value at the moment the `Make Payment` button was clicked
+ - it can be refreshed
+ - warnings are shown if the fee is too Low or to High
+
+### Create PSBT
+ - based on the Inputs & Outputs selected by the user a PSBT will be generated
+ - this wallet is watch-only, therefore does not support signing
+ - it is not mandatory for the `Selected Amount` to be grater than `Payed Amount`
+ - the generated PSBT can be combined with other PSBTs that add more inputs.
+ - the generated PSBT can be imported for signing into different wallets like Electrum
+ - import the PSBT into Electrum and check the In/Outs/Fee (see `screenshot 3`)
+
+## Screensots
+- screenshot 1:
+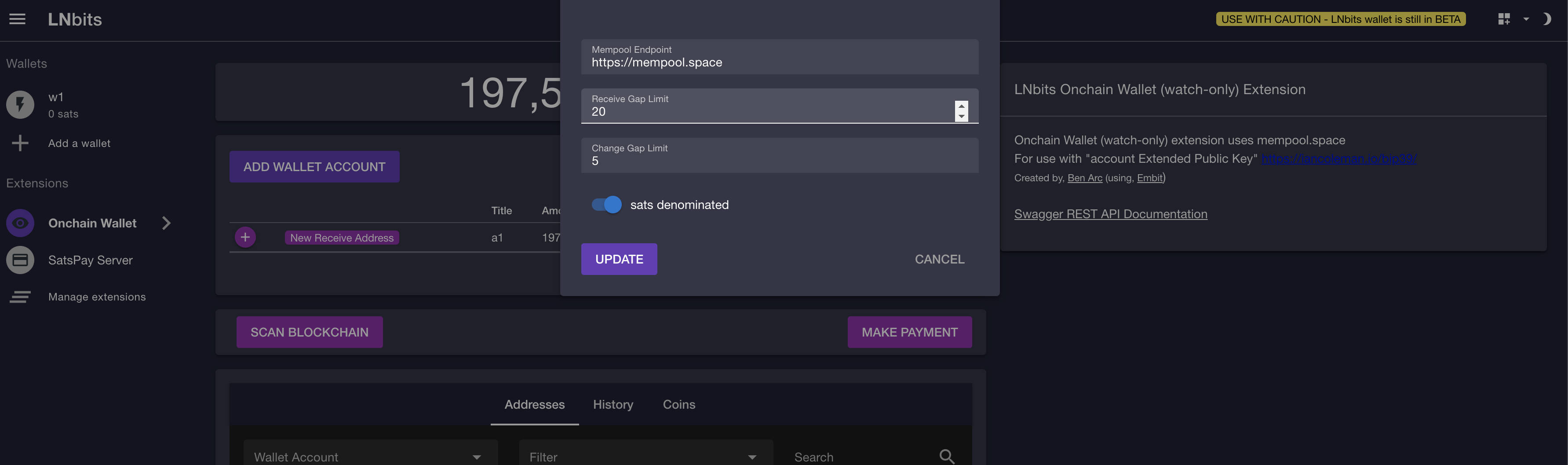
+
+- screenshot 2:
+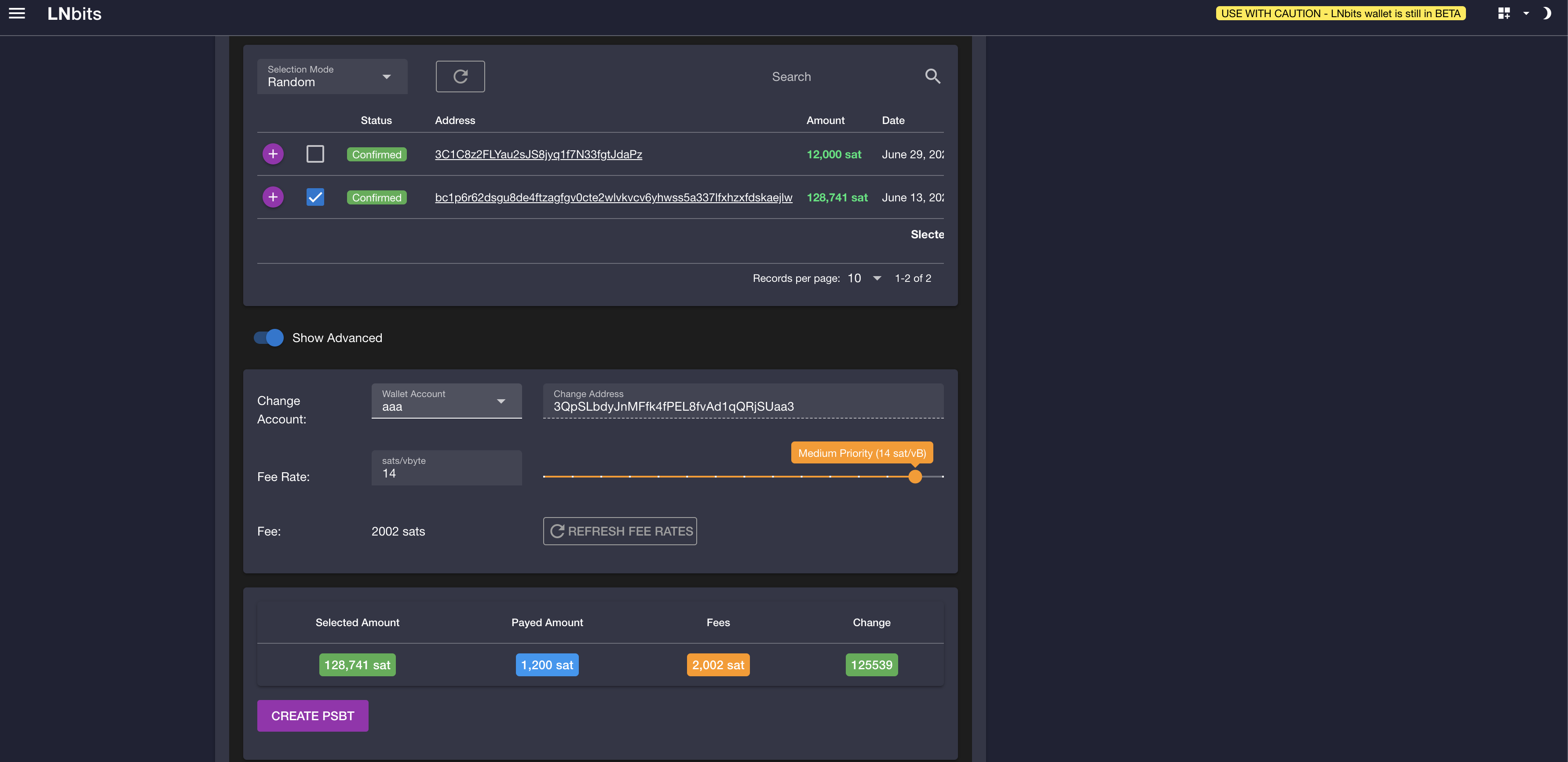
+
+- screenshot 3:
+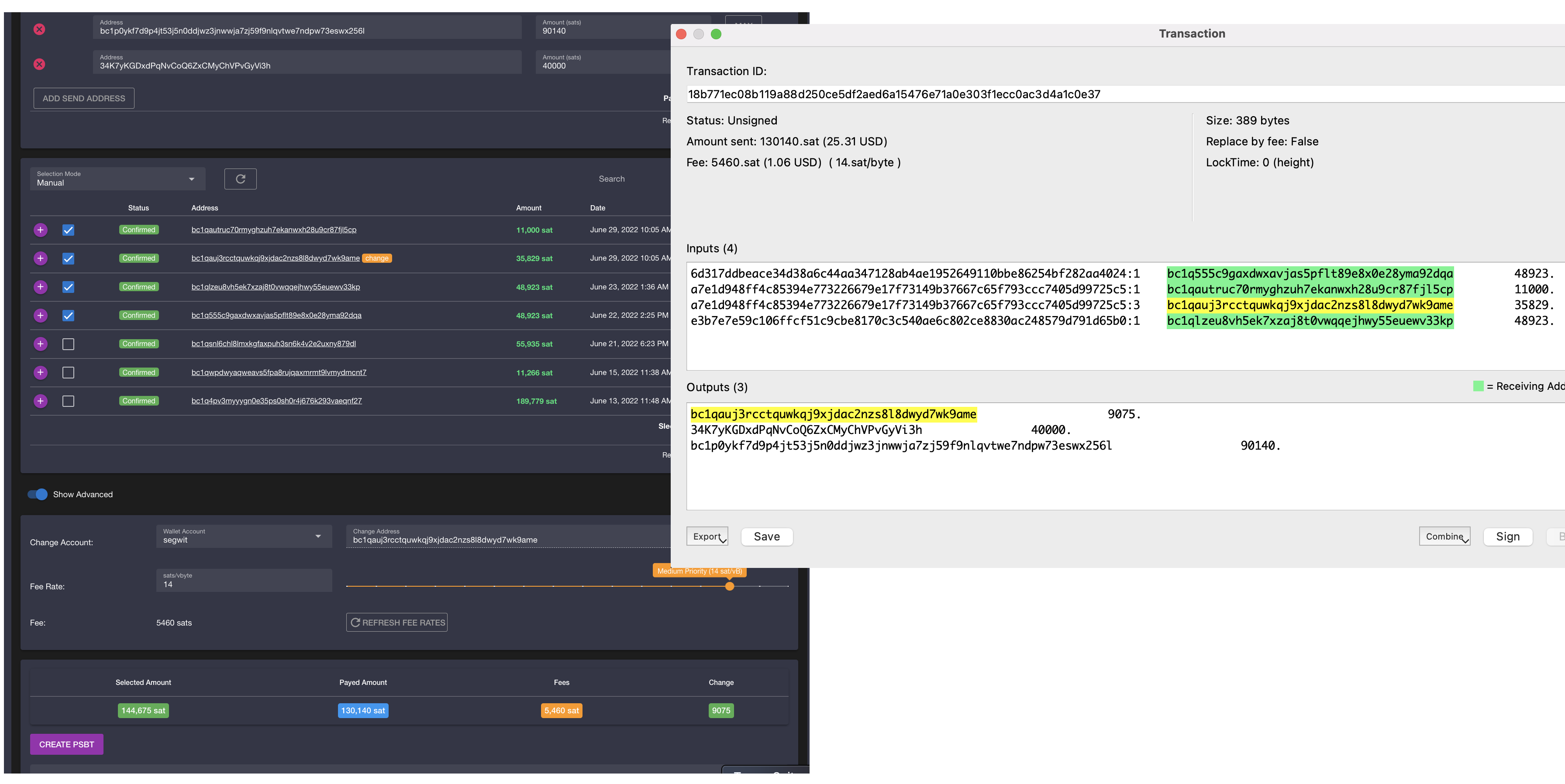
+
+- screenshot 4:
+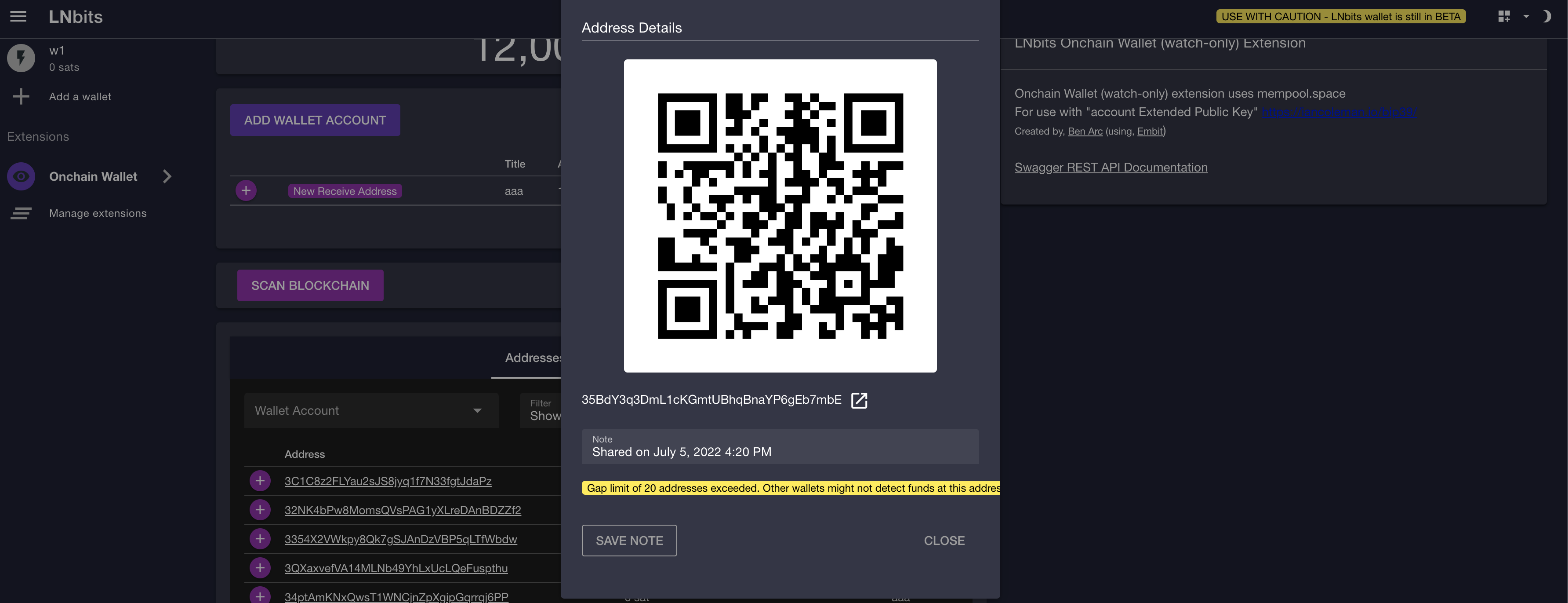
+
+
diff --git a/lnbits/extensions/watchonly/__init__.py b/lnbits/extensions/watchonly/__init__.py
index 0c5259803..a7fff888a 100644
--- a/lnbits/extensions/watchonly/__init__.py
+++ b/lnbits/extensions/watchonly/__init__.py
@@ -1,10 +1,18 @@
from fastapi import APIRouter
+from fastapi.staticfiles import StaticFiles
from lnbits.db import Database
from lnbits.helpers import template_renderer
db = Database("ext_watchonly")
+watchonly_static_files = [
+ {
+ "path": "/watchonly/static",
+ "app": StaticFiles(directory="lnbits/extensions/watchonly/static"),
+ "name": "watchonly_static",
+ }
+]
watchonly_ext: APIRouter = APIRouter(prefix="/watchonly", tags=["watchonly"])
diff --git a/lnbits/extensions/watchonly/config.json b/lnbits/extensions/watchonly/config.json
index 48c19ef07..6331418cd 100644
--- a/lnbits/extensions/watchonly/config.json
+++ b/lnbits/extensions/watchonly/config.json
@@ -1,8 +1,9 @@
{
- "name": "Watch Only",
+ "name": "Onchain Wallet",
"short_description": "Onchain watch only wallets",
"icon": "visibility",
"contributors": [
- "arcbtc"
+ "arcbtc",
+ "motorina0"
]
}
diff --git a/lnbits/extensions/watchonly/crud.py b/lnbits/extensions/watchonly/crud.py
index 0ce3ead9d..b88a7df7e 100644
--- a/lnbits/extensions/watchonly/crud.py
+++ b/lnbits/extensions/watchonly/crud.py
@@ -1,81 +1,16 @@
+import json
from typing import List, Optional
-from embit.descriptor import Descriptor, Key # type: ignore
-from embit.descriptor.arguments import AllowedDerivation # type: ignore
-from embit.networks import NETWORKS # type: ignore
-
from lnbits.helpers import urlsafe_short_hash
from . import db
-from .models import Addresses, Mempool, Wallets
+from .helpers import derive_address, parse_key
+from .models import Address, Config, Mempool, WalletAccount
##########################WALLETS####################
-def detect_network(k):
- version = k.key.version
- for network_name in NETWORKS:
- net = NETWORKS[network_name]
- # not found in this network
- if version in [net["xpub"], net["ypub"], net["zpub"], net["Zpub"], net["Ypub"]]:
- return net
-
-
-def parse_key(masterpub: str):
- """Parses masterpub or descriptor and returns a tuple: (Descriptor, network)
- To create addresses use descriptor.derive(num).address(network=network)
- """
- network = None
- # probably a single key
- if "(" not in masterpub:
- k = Key.from_string(masterpub)
- if not k.is_extended:
- raise ValueError("The key is not a master public key")
- if k.is_private:
- raise ValueError("Private keys are not allowed")
- # check depth
- if k.key.depth != 3:
- raise ValueError(
- "Non-standard depth. Only bip44, bip49 and bip84 are supported with bare xpubs. For custom derivation paths use descriptors."
- )
- # if allowed derivation is not provided use default /{0,1}/*
- if k.allowed_derivation is None:
- k.allowed_derivation = AllowedDerivation.default()
- # get version bytes
- version = k.key.version
- for network_name in NETWORKS:
- net = NETWORKS[network_name]
- # not found in this network
- if version in [net["xpub"], net["ypub"], net["zpub"]]:
- network = net
- if version == net["xpub"]:
- desc = Descriptor.from_string("pkh(%s)" % str(k))
- elif version == net["ypub"]:
- desc = Descriptor.from_string("sh(wpkh(%s))" % str(k))
- elif version == net["zpub"]:
- desc = Descriptor.from_string("wpkh(%s)" % str(k))
- break
- # we didn't find correct version
- if network is None:
- raise ValueError("Unknown master public key version")
- else:
- desc = Descriptor.from_string(masterpub)
- if not desc.is_wildcard:
- raise ValueError("Descriptor should have wildcards")
- for k in desc.keys:
- if k.is_extended:
- net = detect_network(k)
- if net is None:
- raise ValueError(f"Unknown version: {k}")
- if network is not None and network != net:
- raise ValueError("Keys from different networks")
- network = net
- return desc, network
-
-
-async def create_watch_wallet(user: str, masterpub: str, title: str) -> Wallets:
- # check the masterpub is fine, it will raise an exception if not
- parse_key(masterpub)
+async def create_watch_wallet(w: WalletAccount) -> WalletAccount:
wallet_id = urlsafe_short_hash()
await db.execute(
"""
@@ -83,34 +18,44 @@ async def create_watch_wallet(user: str, masterpub: str, title: str) -> Wallets:
id,
"user",
masterpub,
+ fingerprint,
title,
+ type,
address_no,
balance
)
- VALUES (?, ?, ?, ?, ?, ?)
+ VALUES (?, ?, ?, ?, ?, ?, ?, ?)
""",
- # address_no is -1 so fresh address on empty wallet can get address with index 0
- (wallet_id, user, masterpub, title, -1, 0),
+ (
+ wallet_id,
+ w.user,
+ w.masterpub,
+ w.fingerprint,
+ w.title,
+ w.type,
+ w.address_no,
+ w.balance,
+ ),
)
return await get_watch_wallet(wallet_id)
-async def get_watch_wallet(wallet_id: str) -> Optional[Wallets]:
+async def get_watch_wallet(wallet_id: str) -> Optional[WalletAccount]:
row = await db.fetchone(
"SELECT * FROM watchonly.wallets WHERE id = ?", (wallet_id,)
)
- return Wallets.from_row(row) if row else None
+ return WalletAccount.from_row(row) if row else None
-async def get_watch_wallets(user: str) -> List[Wallets]:
+async def get_watch_wallets(user: str) -> List[WalletAccount]:
rows = await db.fetchall(
"""SELECT * FROM watchonly.wallets WHERE "user" = ?""", (user,)
)
- return [Wallets(**row) for row in rows]
+ return [WalletAccount(**row) for row in rows]
-async def update_watch_wallet(wallet_id: str, **kwargs) -> Optional[Wallets]:
+async def update_watch_wallet(wallet_id: str, **kwargs) -> Optional[WalletAccount]:
q = ", ".join([f"{field[0]} = ?" for field in kwargs.items()])
await db.execute(
@@ -119,65 +64,184 @@ async def update_watch_wallet(wallet_id: str, **kwargs) -> Optional[Wallets]:
row = await db.fetchone(
"SELECT * FROM watchonly.wallets WHERE id = ?", (wallet_id,)
)
- return Wallets.from_row(row) if row else None
+ return WalletAccount.from_row(row) if row else None
async def delete_watch_wallet(wallet_id: str) -> None:
await db.execute("DELETE FROM watchonly.wallets WHERE id = ?", (wallet_id,))
- ########################ADDRESSES#######################
+
+########################ADDRESSES#######################
-async def get_derive_address(wallet_id: str, num: int):
- wallet = await get_watch_wallet(wallet_id)
- key = wallet.masterpub
- desc, network = parse_key(key)
- return desc.derive(num).address(network=network)
-
-
-async def get_fresh_address(wallet_id: str) -> Optional[Addresses]:
+async def get_fresh_address(wallet_id: str) -> Optional[Address]:
+ # todo: move logic to views_api after satspay refactoring
wallet = await get_watch_wallet(wallet_id)
if not wallet:
return None
- address = await get_derive_address(wallet_id, wallet.address_no + 1)
+ wallet_addresses = await get_addresses(wallet_id)
+ receive_addresses = list(
+ filter(
+ lambda addr: addr.branch_index == 0 and addr.has_activity, wallet_addresses
+ )
+ )
+ last_receive_index = (
+ receive_addresses.pop().address_index if receive_addresses else -1
+ )
+ address_index = (
+ last_receive_index
+ if last_receive_index > wallet.address_no
+ else wallet.address_no
+ )
- await update_watch_wallet(wallet_id=wallet_id, address_no=wallet.address_no + 1)
- masterpub_id = urlsafe_short_hash()
- await db.execute(
- """
+ address = await get_address_at_index(wallet_id, 0, address_index + 1)
+
+ if not address:
+ addresses = await create_fresh_addresses(
+ wallet_id, address_index + 1, address_index + 2
+ )
+ address = addresses.pop()
+
+ await update_watch_wallet(wallet_id, **{"address_no": address_index + 1})
+
+ return address
+
+
+async def create_fresh_addresses(
+ wallet_id: str,
+ start_address_index: int,
+ end_address_index: int,
+ change_address=False,
+) -> List[Address]:
+ if start_address_index > end_address_index:
+ return None
+
+ wallet = await get_watch_wallet(wallet_id)
+ if not wallet:
+ return None
+
+ branch_index = 1 if change_address else 0
+
+ for address_index in range(start_address_index, end_address_index):
+ address = await derive_address(wallet.masterpub, address_index, branch_index)
+
+ await db.execute(
+ """
INSERT INTO watchonly.addresses (
id,
address,
wallet,
- amount
+ amount,
+ branch_index,
+ address_index
)
- VALUES (?, ?, ?, ?)
+ VALUES (?, ?, ?, ?, ?, ?)
""",
- (masterpub_id, address, wallet_id, 0),
+ (urlsafe_short_hash(), address, wallet_id, 0, branch_index, address_index),
+ )
+
+ # return fresh addresses
+ rows = await db.fetchall(
+ """
+ SELECT * FROM watchonly.addresses
+ WHERE wallet = ? AND branch_index = ? AND address_index >= ? AND address_index < ?
+ ORDER BY branch_index, address_index
+ """,
+ (wallet_id, branch_index, start_address_index, end_address_index),
)
- return await get_address(address)
+ return [Address(**row) for row in rows]
-async def get_address(address: str) -> Optional[Addresses]:
+async def get_address(address: str) -> Optional[Address]:
row = await db.fetchone(
"SELECT * FROM watchonly.addresses WHERE address = ?", (address,)
)
- return Addresses.from_row(row) if row else None
+ return Address.from_row(row) if row else None
-async def get_addresses(wallet_id: str) -> List[Addresses]:
- rows = await db.fetchall(
- "SELECT * FROM watchonly.addresses WHERE wallet = ?", (wallet_id,)
+async def get_address_at_index(
+ wallet_id: str, branch_index: int, address_index: int
+) -> Optional[Address]:
+ row = await db.fetchone(
+ """
+ SELECT * FROM watchonly.addresses
+ WHERE wallet = ? AND branch_index = ? AND address_index = ?
+ """,
+ (
+ wallet_id,
+ branch_index,
+ address_index,
+ ),
)
- return [Addresses(**row) for row in rows]
+ return Address.from_row(row) if row else None
+
+
+async def get_addresses(wallet_id: str) -> List[Address]:
+ rows = await db.fetchall(
+ """
+ SELECT * FROM watchonly.addresses WHERE wallet = ?
+ ORDER BY branch_index, address_index
+ """,
+ (wallet_id,),
+ )
+
+ return [Address(**row) for row in rows]
+
+
+async def update_address(id: str, **kwargs) -> Optional[Address]:
+ q = ", ".join([f"{field[0]} = ?" for field in kwargs.items()])
+
+ await db.execute(
+ f"""UPDATE watchonly.addresses SET {q} WHERE id = ? """,
+ (*kwargs.values(), id),
+ )
+ row = await db.fetchone("SELECT * FROM watchonly.addresses WHERE id = ?", (id))
+ return Address.from_row(row) if row else None
+
+
+async def delete_addresses_for_wallet(wallet_id: str) -> None:
+ await db.execute("DELETE FROM watchonly.addresses WHERE wallet = ?", (wallet_id,))
+
+
+######################CONFIG#######################
+async def create_config(user: str) -> Config:
+ config = Config()
+ await db.execute(
+ """
+ INSERT INTO watchonly.config ("user", json_data)
+ VALUES (?, ?)
+ """,
+ (user, json.dumps(config.dict())),
+ )
+ row = await db.fetchone(
+ """SELECT json_data FROM watchonly.config WHERE "user" = ?""", (user,)
+ )
+ return json.loads(row[0], object_hook=lambda d: Config(**d))
+
+
+async def update_config(config: Config, user: str) -> Optional[Config]:
+ await db.execute(
+ f"""UPDATE watchonly.config SET json_data = ? WHERE "user" = ?""",
+ (json.dumps(config.dict()), user),
+ )
+ row = await db.fetchone(
+ """SELECT json_data FROM watchonly.config WHERE "user" = ?""", (user,)
+ )
+ return json.loads(row[0], object_hook=lambda d: Config(**d))
+
+
+async def get_config(user: str) -> Optional[Config]:
+ row = await db.fetchone(
+ """SELECT json_data FROM watchonly.config WHERE "user" = ?""", (user,)
+ )
+ return json.loads(row[0], object_hook=lambda d: Config(**d)) if row else None
######################MEMPOOL#######################
-
-
+### TODO: fix statspay dependcy and remove
async def create_mempool(user: str) -> Optional[Mempool]:
await db.execute(
"""
@@ -192,6 +256,7 @@ async def create_mempool(user: str) -> Optional[Mempool]:
return Mempool.from_row(row) if row else None
+### TODO: fix statspay dependcy and remove
async def update_mempool(user: str, **kwargs) -> Optional[Mempool]:
q = ", ".join([f"{field[0]} = ?" for field in kwargs.items()])
@@ -205,6 +270,7 @@ async def update_mempool(user: str, **kwargs) -> Optional[Mempool]:
return Mempool.from_row(row) if row else None
+### TODO: fix statspay dependcy and remove
async def get_mempool(user: str) -> Mempool:
row = await db.fetchone(
"""SELECT * FROM watchonly.mempool WHERE "user" = ?""", (user,)
diff --git a/lnbits/extensions/watchonly/helpers.py b/lnbits/extensions/watchonly/helpers.py
new file mode 100644
index 000000000..74125dde8
--- /dev/null
+++ b/lnbits/extensions/watchonly/helpers.py
@@ -0,0 +1,69 @@
+from embit.descriptor import Descriptor, Key # type: ignore
+from embit.descriptor.arguments import AllowedDerivation # type: ignore
+from embit.networks import NETWORKS # type: ignore
+
+
+def detect_network(k):
+ version = k.key.version
+ for network_name in NETWORKS:
+ net = NETWORKS[network_name]
+ # not found in this network
+ if version in [net["xpub"], net["ypub"], net["zpub"], net["Zpub"], net["Ypub"]]:
+ return net
+
+
+def parse_key(masterpub: str) -> Descriptor:
+ """Parses masterpub or descriptor and returns a tuple: (Descriptor, network)
+ To create addresses use descriptor.derive(num).address(network=network)
+ """
+ network = None
+ # probably a single key
+ if "(" not in masterpub:
+ k = Key.from_string(masterpub)
+ if not k.is_extended:
+ raise ValueError("The key is not a master public key")
+ if k.is_private:
+ raise ValueError("Private keys are not allowed")
+ # check depth
+ if k.key.depth != 3:
+ raise ValueError(
+ "Non-standard depth. Only bip44, bip49 and bip84 are supported with bare xpubs. For custom derivation paths use descriptors."
+ )
+ # if allowed derivation is not provided use default /{0,1}/*
+ if k.allowed_derivation is None:
+ k.allowed_derivation = AllowedDerivation.default()
+ # get version bytes
+ version = k.key.version
+ for network_name in NETWORKS:
+ net = NETWORKS[network_name]
+ # not found in this network
+ if version in [net["xpub"], net["ypub"], net["zpub"]]:
+ network = net
+ if version == net["xpub"]:
+ desc = Descriptor.from_string("pkh(%s)" % str(k))
+ elif version == net["ypub"]:
+ desc = Descriptor.from_string("sh(wpkh(%s))" % str(k))
+ elif version == net["zpub"]:
+ desc = Descriptor.from_string("wpkh(%s)" % str(k))
+ break
+ # we didn't find correct version
+ if network is None:
+ raise ValueError("Unknown master public key version")
+ else:
+ desc = Descriptor.from_string(masterpub)
+ if not desc.is_wildcard:
+ raise ValueError("Descriptor should have wildcards")
+ for k in desc.keys:
+ if k.is_extended:
+ net = detect_network(k)
+ if net is None:
+ raise ValueError(f"Unknown version: {k}")
+ if network is not None and network != net:
+ raise ValueError("Keys from different networks")
+ network = net
+ return desc, network
+
+
+async def derive_address(masterpub: str, num: int, branch_index=0):
+ desc, network = parse_key(masterpub)
+ return desc.derive(num, branch_index).address(network=network)
diff --git a/lnbits/extensions/watchonly/migrations.py b/lnbits/extensions/watchonly/migrations.py
index 05c229b53..8e371a2a0 100644
--- a/lnbits/extensions/watchonly/migrations.py
+++ b/lnbits/extensions/watchonly/migrations.py
@@ -34,3 +34,50 @@ async def m001_initial(db):
);
"""
)
+
+
+async def m002_add_columns_to_adresses(db):
+ """
+ Add 'branch_index', 'address_index', 'has_activity' and 'note' columns to the 'addresses' table
+ """
+
+ await db.execute(
+ "ALTER TABLE watchonly.addresses ADD COLUMN branch_index INTEGER NOT NULL DEFAULT 0;"
+ )
+ await db.execute(
+ "ALTER TABLE watchonly.addresses ADD COLUMN address_index INTEGER NOT NULL DEFAULT 0;"
+ )
+ await db.execute(
+ "ALTER TABLE watchonly.addresses ADD COLUMN has_activity BOOLEAN DEFAULT false;"
+ )
+ await db.execute("ALTER TABLE watchonly.addresses ADD COLUMN note TEXT;")
+
+
+async def m003_add_columns_to_wallets(db):
+ """
+ Add 'type' and 'fingerprint' columns to the 'wallets' table
+ """
+
+ await db.execute("ALTER TABLE watchonly.wallets ADD COLUMN type TEXT;")
+ await db.execute(
+ "ALTER TABLE watchonly.wallets ADD COLUMN fingerprint TEXT NOT NULL DEFAULT '';"
+ )
+
+
+async def m004_create_config_table(db):
+ """
+ Allow the extension to persist and retrieve any number of config values.
+ Each user has its configurations saved as a JSON string
+ """
+
+ await db.execute(
+ """CREATE TABLE watchonly.config (
+ "user" TEXT NOT NULL,
+ json_data TEXT NOT NULL
+ );"""
+ )
+
+ ### TODO: fix statspay dependcy first
+ # await db.execute(
+ # "DROP TABLE watchonly.wallets;"
+ # )
diff --git a/lnbits/extensions/watchonly/models.py b/lnbits/extensions/watchonly/models.py
index d0894097f..bc10e4210 100644
--- a/lnbits/extensions/watchonly/models.py
+++ b/lnbits/extensions/watchonly/models.py
@@ -1,4 +1,5 @@
from sqlite3 import Row
+from typing import List
from fastapi.param_functions import Query
from pydantic import BaseModel
@@ -9,19 +10,22 @@ class CreateWallet(BaseModel):
title: str = Query("")
-class Wallets(BaseModel):
+class WalletAccount(BaseModel):
id: str
user: str
masterpub: str
+ fingerprint: str
title: str
address_no: int
balance: int
+ type: str = ""
@classmethod
- def from_row(cls, row: Row) -> "Wallets":
+ def from_row(cls, row: Row) -> "WalletAccount":
return cls(**dict(row))
+### TODO: fix statspay dependcy and remove
class Mempool(BaseModel):
user: str
endpoint: str
@@ -31,12 +35,55 @@ class Mempool(BaseModel):
return cls(**dict(row))
-class Addresses(BaseModel):
+class Address(BaseModel):
id: str
address: str
wallet: str
- amount: int
+ amount: int = 0
+ branch_index: int = 0
+ address_index: int
+ note: str = None
+ has_activity: bool = False
@classmethod
- def from_row(cls, row: Row) -> "Addresses":
+ def from_row(cls, row: Row) -> "Address":
return cls(**dict(row))
+
+
+class TransactionInput(BaseModel):
+ tx_id: str
+ vout: int
+ amount: int
+ address: str
+ branch_index: int
+ address_index: int
+ masterpub_fingerprint: str
+ tx_hex: str
+
+
+class TransactionOutput(BaseModel):
+ amount: int
+ address: str
+ branch_index: int = None
+ address_index: int = None
+ masterpub_fingerprint: str = None
+
+
+class MasterPublicKey(BaseModel):
+ public_key: str
+ fingerprint: str
+
+
+class CreatePsbt(BaseModel):
+ masterpubs: List[MasterPublicKey]
+ inputs: List[TransactionInput]
+ outputs: List[TransactionOutput]
+ fee_rate: int
+ tx_size: int
+
+
+class Config(BaseModel):
+ mempool_endpoint = "https://mempool.space"
+ receive_gap_limit = 20
+ change_gap_limit = 5
+ sats_denominated = True
diff --git a/lnbits/extensions/watchonly/static/js/index.js b/lnbits/extensions/watchonly/static/js/index.js
new file mode 100644
index 000000000..5eee21761
--- /dev/null
+++ b/lnbits/extensions/watchonly/static/js/index.js
@@ -0,0 +1,735 @@
+Vue.component(VueQrcode.name, VueQrcode)
+
+Vue.filter('reverse', function (value) {
+ // slice to make a copy of array, then reverse the copy
+ return value.slice().reverse()
+})
+
+new Vue({
+ el: '#vue',
+ mixins: [windowMixin],
+ data: function () {
+ return {
+ DUST_LIMIT: 546,
+ filter: '',
+
+ scan: {
+ scanning: false,
+ scanCount: 0,
+ scanIndex: 0
+ },
+
+ currentAddress: null,
+
+ tab: 'addresses',
+
+ config: {
+ data: {
+ mempool_endpoint: 'https://mempool.space',
+ receive_gap_limit: 20,
+ change_gap_limit: 5
+ },
+ DEFAULT_RECEIVE_GAP_LIMIT: 20,
+ show: false
+ },
+
+ formDialog: {
+ show: false,
+ data: {}
+ },
+
+ qrCodeDialog: {
+ show: false,
+ data: null
+ },
+ ...tables,
+ ...tableData
+ }
+ },
+
+ methods: {
+ //################### CONFIG ###################
+ getConfig: async function () {
+ try {
+ const {data} = await LNbits.api.request(
+ 'GET',
+ '/watchonly/api/v1/config',
+ this.g.user.wallets[0].adminkey
+ )
+ this.config.data = data
+ } catch (error) {
+ LNbits.utils.notifyApiError(error)
+ }
+ },
+ updateConfig: async function () {
+ const wallet = this.g.user.wallets[0]
+ try {
+ await LNbits.api.request(
+ 'PUT',
+ '/watchonly/api/v1/config',
+ wallet.adminkey,
+ this.config.data
+ )
+ this.config.show = false
+ } catch (error) {
+ LNbits.utils.notifyApiError(error)
+ }
+ },
+
+ //################### WALLETS ###################
+ getWalletName: function (walletId) {
+ const wallet = this.walletAccounts.find(wl => wl.id === walletId)
+ return wallet ? wallet.title : 'unknown'
+ },
+ addWalletAccount: async function () {
+ const wallet = this.g.user.wallets[0]
+ const data = _.omit(this.formDialog.data, 'wallet')
+ await this.createWalletAccount(wallet, data)
+ },
+ createWalletAccount: async function (wallet, data) {
+ try {
+ const response = await LNbits.api.request(
+ 'POST',
+ '/watchonly/api/v1/wallet',
+ wallet.adminkey,
+ data
+ )
+ this.walletAccounts.push(mapWalletAccount(response.data))
+ this.formDialog.show = false
+
+ await this.refreshWalletAccounts()
+ await this.refreshAddresses()
+
+ if (!this.payment.changeWallett) {
+ this.payment.changeWallet = this.walletAccounts[0]
+ this.selectChangeAddress(this.payment.changeWallet)
+ }
+ } catch (error) {
+ LNbits.utils.notifyApiError(error)
+ }
+ },
+ deleteWalletAccount: function (walletAccountId) {
+ LNbits.utils
+ .confirmDialog(
+ 'Are you sure you want to delete this watch only wallet?'
+ )
+ .onOk(async () => {
+ try {
+ await LNbits.api.request(
+ 'DELETE',
+ '/watchonly/api/v1/wallet/' + walletAccountId,
+ this.g.user.wallets[0].adminkey
+ )
+ this.walletAccounts = _.reject(this.walletAccounts, function (obj) {
+ return obj.id === walletAccountId
+ })
+ await this.refreshWalletAccounts()
+ await this.refreshAddresses()
+ if (
+ this.payment.changeWallet &&
+ this.payment.changeWallet.id === walletAccountId
+ ) {
+ this.payment.changeWallet = this.walletAccounts[0]
+ this.selectChangeAddress(this.payment.changeWallet)
+ }
+ await this.scanAddressWithAmount()
+ } catch (error) {
+ this.$q.notify({
+ type: 'warning',
+ message: 'Error while deleting wallet account. Please try again.',
+ timeout: 10000
+ })
+ }
+ })
+ },
+ getAddressesForWallet: async function (walletId) {
+ try {
+ const {data} = await LNbits.api.request(
+ 'GET',
+ '/watchonly/api/v1/addresses/' + walletId,
+ this.g.user.wallets[0].inkey
+ )
+ return data.map(mapAddressesData)
+ } catch (err) {
+ this.$q.notify({
+ type: 'warning',
+ message: `Failed to fetch addresses for wallet with id ${walletId}.`,
+ timeout: 10000
+ })
+ LNbits.utils.notifyApiError(err)
+ }
+ return []
+ },
+ getWatchOnlyWallets: async function () {
+ try {
+ const {data} = await LNbits.api.request(
+ 'GET',
+ '/watchonly/api/v1/wallet',
+ this.g.user.wallets[0].inkey
+ )
+ return data
+ } catch (error) {
+ this.$q.notify({
+ type: 'warning',
+ message: 'Failed to fetch wallets.',
+ timeout: 10000
+ })
+ LNbits.utils.notifyApiError(error)
+ }
+ return []
+ },
+ refreshWalletAccounts: async function () {
+ const wallets = await this.getWatchOnlyWallets()
+ this.walletAccounts = wallets.map(w => mapWalletAccount(w))
+ },
+ getAmmountForWallet: function (walletId) {
+ const amount = this.addresses.data
+ .filter(a => a.wallet === walletId)
+ .reduce((t, a) => t + a.amount || 0, 0)
+ return this.satBtc(amount)
+ },
+
+ //################### ADDRESSES ###################
+
+ refreshAddresses: async function () {
+ const wallets = await this.getWatchOnlyWallets()
+ this.addresses.data = []
+ for (const {id, type} of wallets) {
+ const newAddresses = await this.getAddressesForWallet(id)
+ const uniqueAddresses = newAddresses.filter(
+ newAddr =>
+ !this.addresses.data.find(a => a.address === newAddr.address)
+ )
+
+ const lastAcctiveAddress =
+ uniqueAddresses.filter(a => !a.isChange && a.hasActivity).pop() || {}
+
+ uniqueAddresses.forEach(a => {
+ a.expanded = false
+ a.accountType = type
+ a.gapLimitExceeded =
+ !a.isChange &&
+ a.addressIndex >
+ lastAcctiveAddress.addressIndex +
+ this.config.DEFAULT_RECEIVE_GAP_LIMIT
+ })
+ this.addresses.data.push(...uniqueAddresses)
+ }
+ },
+ updateAmountForAddress: async function (addressData, amount = 0) {
+ try {
+ const wallet = this.g.user.wallets[0]
+ addressData.amount = amount
+ if (!addressData.isChange) {
+ const addressWallet = this.walletAccounts.find(
+ w => w.id === addressData.wallet
+ )
+ if (
+ addressWallet &&
+ addressWallet.address_no < addressData.addressIndex
+ ) {
+ addressWallet.address_no = addressData.addressIndex
+ }
+ }
+
+ await LNbits.api.request(
+ 'PUT',
+ `/watchonly/api/v1/address/${addressData.id}`,
+ wallet.adminkey,
+ {amount}
+ )
+ } catch (err) {
+ addressData.error = 'Failed to refresh amount for address'
+ this.$q.notify({
+ type: 'warning',
+ message: `Failed to refresh amount for address ${addressData.address}`,
+ timeout: 10000
+ })
+ LNbits.utils.notifyApiError(err)
+ }
+ },
+ updateNoteForAddress: async function (addressData, note) {
+ try {
+ const wallet = this.g.user.wallets[0]
+ await LNbits.api.request(
+ 'PUT',
+ `/watchonly/api/v1/address/${addressData.id}`,
+ wallet.adminkey,
+ {note: addressData.note}
+ )
+ const updatedAddress =
+ this.addresses.data.find(a => a.id === addressData.id) || {}
+ updatedAddress.note = note
+ } catch (err) {
+ LNbits.utils.notifyApiError(err)
+ }
+ },
+ getFilteredAddresses: function () {
+ const selectedWalletId = this.addresses.selectedWallet?.id
+ const filter = this.addresses.filterValues || []
+ const includeChangeAddrs = filter.includes('Show Change Addresses')
+ const includeGapAddrs = filter.includes('Show Gap Addresses')
+ const excludeNoAmount = filter.includes('Only With Amount')
+
+ const walletsLimit = this.walletAccounts.reduce((r, w) => {
+ r[`_${w.id}`] = w.address_no
+ return r
+ }, {})
+
+ const addresses = this.addresses.data.filter(
+ a =>
+ (includeChangeAddrs || !a.isChange) &&
+ (includeGapAddrs ||
+ a.isChange ||
+ a.addressIndex <= walletsLimit[`_${a.wallet}`]) &&
+ !(excludeNoAmount && a.amount === 0) &&
+ (!selectedWalletId || a.wallet === selectedWalletId)
+ )
+ return addresses
+ },
+ openGetFreshAddressDialog: async function (walletId) {
+ const {data} = await LNbits.api.request(
+ 'GET',
+ `/watchonly/api/v1/address/${walletId}`,
+ this.g.user.wallets[0].inkey
+ )
+ const addressData = mapAddressesData(data)
+
+ addressData.note = `Shared on ${currentDateTime()}`
+ const lastAcctiveAddress =
+ this.addresses.data
+ .filter(
+ a => a.wallet === addressData.wallet && !a.isChange && a.hasActivity
+ )
+ .pop() || {}
+ addressData.gapLimitExceeded =
+ !addressData.isChange &&
+ addressData.addressIndex >
+ lastAcctiveAddress.addressIndex +
+ this.config.DEFAULT_RECEIVE_GAP_LIMIT
+
+ this.openQrCodeDialog(addressData)
+ const wallet = this.walletAccounts.find(w => w.id === walletId) || {}
+ wallet.address_no = addressData.addressIndex
+ await this.refreshAddresses()
+ },
+
+ //################### ADDRESS HISTORY ###################
+ addressHistoryFromTxs: function (addressData, txs) {
+ const addressHistory = []
+ txs.forEach(tx => {
+ const sent = tx.vin
+ .filter(
+ vin => vin.prevout.scriptpubkey_address === addressData.address
+ )
+ .map(vin => mapInputToSentHistory(tx, addressData, vin))
+
+ const received = tx.vout
+ .filter(vout => vout.scriptpubkey_address === addressData.address)
+ .map(vout => mapOutputToReceiveHistory(tx, addressData, vout))
+ addressHistory.push(...sent, ...received)
+ })
+ return addressHistory
+ },
+ getFilteredAddressesHistory: function () {
+ return this.addresses.history.filter(
+ a => (!a.isChange || a.sent) && !a.isSubItem
+ )
+ },
+ exportHistoryToCSV: function () {
+ const history = this.getFilteredAddressesHistory().map(a => ({
+ ...a,
+ action: a.sent ? 'Sent' : 'Received'
+ }))
+ LNbits.utils.exportCSV(
+ this.historyTable.exportColums,
+ history,
+ 'address-history'
+ )
+ },
+ markSameTxAddressHistory: function () {
+ this.addresses.history
+ .filter(s => s.sent)
+ .forEach((el, i, arr) => {
+ if (el.isSubItem) return
+
+ const sameTxItems = arr.slice(i + 1).filter(e => e.txId === el.txId)
+ if (!sameTxItems.length) return
+ sameTxItems.forEach(e => {
+ e.isSubItem = true
+ })
+
+ el.totalAmount =
+ el.amount + sameTxItems.reduce((t, e) => (t += e.amount || 0), 0)
+ el.sameTxItems = sameTxItems
+ })
+ },
+ showAddressHistoryDetails: function (addressHistory) {
+ addressHistory.expanded = true
+ },
+
+ //################### PAYMENT ###################
+ createTx: function (excludeChange = false) {
+ const tx = {
+ fee_rate: this.payment.feeRate,
+ tx_size: this.payment.txSize,
+ masterpubs: this.walletAccounts.map(w => ({
+ public_key: w.masterpub,
+ fingerprint: w.fingerprint
+ }))
+ }
+ tx.inputs = this.utxos.data
+ .filter(utxo => utxo.selected)
+ .map(mapUtxoToPsbtInput)
+ .sort((a, b) =>
+ a.tx_id < b.tx_id ? -1 : a.tx_id > b.tx_id ? 1 : a.vout - b.vout
+ )
+
+ tx.outputs = this.payment.data.map(out => ({
+ address: out.address,
+ amount: out.amount
+ }))
+
+ if (excludeChange) {
+ this.payment.changeAmount = 0
+ } else {
+ const change = this.createChangeOutput()
+ this.payment.changeAmount = change.amount
+ if (change.amount >= this.DUST_LIMIT) {
+ tx.outputs.push(change)
+ }
+ }
+ // Only sort by amount on UI level (no lib for address decode)
+ // Should sort by scriptPubKey (as byte array) on the backend
+ tx.outputs.sort((a, b) => a.amount - b.amount)
+
+ return tx
+ },
+ createChangeOutput: function () {
+ const change = this.payment.changeAddress
+ const fee = this.payment.feeRate * this.payment.txSize
+ const inputAmount = this.getTotalSelectedUtxoAmount()
+ const payedAmount = this.getTotalPaymentAmount()
+ const walletAcount =
+ this.walletAccounts.find(w => w.id === change.wallet) || {}
+
+ return {
+ address: change.address,
+ amount: inputAmount - payedAmount - fee,
+ addressIndex: change.addressIndex,
+ addressIndex: change.addressIndex,
+ masterpub_fingerprint: walletAcount.fingerprint
+ }
+ },
+ computeFee: function () {
+ const tx = this.createTx()
+ this.payment.txSize = Math.round(txSize(tx))
+ return this.payment.feeRate * this.payment.txSize
+ },
+ createPsbt: async function () {
+ const wallet = this.g.user.wallets[0]
+ try {
+ this.computeFee()
+ const tx = this.createTx()
+ txSize(tx)
+ for (const input of tx.inputs) {
+ input.tx_hex = await this.fetchTxHex(input.tx_id)
+ }
+
+ const {data} = await LNbits.api.request(
+ 'POST',
+ '/watchonly/api/v1/psbt',
+ wallet.adminkey,
+ tx
+ )
+
+ this.payment.psbtBase64 = data
+ } catch (err) {
+ LNbits.utils.notifyApiError(err)
+ }
+ },
+ deletePaymentAddress: function (v) {
+ const index = this.payment.data.indexOf(v)
+ if (index !== -1) {
+ this.payment.data.splice(index, 1)
+ }
+ },
+ initPaymentData: async function () {
+ if (!this.payment.show) return
+ await this.refreshAddresses()
+
+ this.payment.showAdvanced = false
+ this.payment.changeWallet = this.walletAccounts[0]
+ this.selectChangeAddress(this.payment.changeWallet)
+
+ await this.refreshRecommendedFees()
+ this.payment.feeRate = this.payment.recommededFees.halfHourFee
+ },
+ getFeeRateLabel: function (feeRate) {
+ const fees = this.payment.recommededFees
+ if (feeRate >= fees.fastestFee) return `High Priority (${feeRate} sat/vB)`
+ if (feeRate >= fees.halfHourFee)
+ return `Medium Priority (${feeRate} sat/vB)`
+ if (feeRate >= fees.hourFee) return `Low Priority (${feeRate} sat/vB)`
+ return `No Priority (${feeRate} sat/vB)`
+ },
+ addPaymentAddress: function () {
+ this.payment.data.push({address: '', amount: undefined})
+ },
+ getTotalPaymentAmount: function () {
+ return this.payment.data.reduce((t, a) => t + (a.amount || 0), 0)
+ },
+ selectChangeAddress: function (wallet = {}) {
+ this.payment.changeAddress =
+ this.addresses.data.find(
+ a => a.wallet === wallet.id && a.isChange && !a.hasActivity
+ ) || {}
+ },
+ goToPaymentView: async function () {
+ this.payment.show = true
+ this.tab = 'utxos'
+ await this.initPaymentData()
+ },
+ sendMaxToAddress: function (paymentAddress = {}) {
+ paymentAddress.amount = 0
+ const tx = this.createTx(true)
+ this.payment.txSize = Math.round(txSize(tx))
+ const fee = this.payment.feeRate * this.payment.txSize
+ const inputAmount = this.getTotalSelectedUtxoAmount()
+ const payedAmount = this.getTotalPaymentAmount()
+ paymentAddress.amount = Math.max(0, inputAmount - payedAmount - fee)
+ },
+
+ //################### UTXOs ###################
+ scanAllAddresses: async function () {
+ await this.refreshAddresses()
+ this.addresses.history = []
+ let addresses = this.addresses.data
+ this.utxos.data = []
+ this.utxos.total = 0
+ // Loop while new funds are found on the gap adresses.
+ // Use 1000 limit as a safety check (scan 20 000 addresses max)
+ for (let i = 0; i < 1000 && addresses.length; i++) {
+ await this.updateUtxosForAddresses(addresses)
+ const oldAddresses = this.addresses.data.slice()
+ await this.refreshAddresses()
+ const newAddresses = this.addresses.data.slice()
+ // check if gap addresses have been extended
+ addresses = newAddresses.filter(
+ newAddr => !oldAddresses.find(oldAddr => oldAddr.id === newAddr.id)
+ )
+ if (addresses.length) {
+ this.$q.notify({
+ type: 'positive',
+ message: 'Funds found! Scanning for more...',
+ timeout: 10000
+ })
+ }
+ }
+ },
+ scanAddressWithAmount: async function () {
+ this.utxos.data = []
+ this.utxos.total = 0
+ this.addresses.history = []
+ const addresses = this.addresses.data.filter(a => a.hasActivity)
+ await this.updateUtxosForAddresses(addresses)
+ },
+ scanAddress: async function (addressData) {
+ this.updateUtxosForAddresses([addressData])
+ this.$q.notify({
+ type: 'positive',
+ message: 'Address Rescanned',
+ timeout: 10000
+ })
+ },
+ updateUtxosForAddresses: async function (addresses = []) {
+ this.scan = {scanning: true, scanCount: addresses.length, scanIndex: 0}
+
+ try {
+ for (addrData of addresses) {
+ const addressHistory = await this.getAddressTxsDelayed(addrData)
+ // remove old entries
+ this.addresses.history = this.addresses.history.filter(
+ h => h.address !== addrData.address
+ )
+
+ // add new entrie
+ this.addresses.history.push(...addressHistory)
+ this.addresses.history.sort((a, b) =>
+ !a.height ? -1 : b.height - a.height
+ )
+ this.markSameTxAddressHistory()
+
+ if (addressHistory.length) {
+ // search only if it ever had any activity
+ const utxos = await this.getAddressTxsUtxoDelayed(addrData.address)
+ this.updateUtxosForAddress(addrData, utxos)
+ }
+
+ this.scan.scanIndex++
+ }
+ } catch (error) {
+ console.error(error)
+ this.$q.notify({
+ type: 'warning',
+ message: 'Failed to scan addresses',
+ timeout: 10000
+ })
+ } finally {
+ this.scan.scanning = false
+ }
+ },
+ updateUtxosForAddress: function (addressData, utxos = []) {
+ const wallet =
+ this.walletAccounts.find(w => w.id === addressData.wallet) || {}
+
+ const newUtxos = utxos.map(utxo =>
+ mapAddressDataToUtxo(wallet, addressData, utxo)
+ )
+ // remove old utxos
+ this.utxos.data = this.utxos.data.filter(
+ u => u.address !== addressData.address
+ )
+ // add new utxos
+ this.utxos.data.push(...newUtxos)
+ if (utxos.length) {
+ this.utxos.data.sort((a, b) => b.sort - a.sort)
+ this.utxos.total = this.utxos.data.reduce(
+ (total, y) => (total += y?.amount || 0),
+ 0
+ )
+ }
+ const addressTotal = utxos.reduce(
+ (total, y) => (total += y?.value || 0),
+ 0
+ )
+ this.updateAmountForAddress(addressData, addressTotal)
+ },
+ getTotalSelectedUtxoAmount: function () {
+ const total = this.utxos.data
+ .filter(u => u.selected)
+ .reduce((t, a) => t + (a.amount || 0), 0)
+ return total
+ },
+ applyUtxoSelectionMode: function () {
+ const payedAmount = this.getTotalPaymentAmount()
+ const mode = this.payment.utxoSelectionMode
+ this.utxos.data.forEach(u => (u.selected = false))
+ const isManual = mode === 'Manual'
+ if (isManual || !payedAmount) return
+
+ const isSelectAll = mode === 'Select All'
+ if (isSelectAll || payedAmount >= this.utxos.total) {
+ this.utxos.data.forEach(u => (u.selected = true))
+ return
+ }
+ const isSmallerFirst = mode === 'Smaller Inputs First'
+ const isLargerFirst = mode === 'Larger Inputs First'
+
+ let selectedUtxos = this.utxos.data.slice()
+ if (isSmallerFirst || isLargerFirst) {
+ const sortFn = isSmallerFirst
+ ? (a, b) => a.amount - b.amount
+ : (a, b) => b.amount - a.amount
+ selectedUtxos.sort(sortFn)
+ } else {
+ // default to random order
+ selectedUtxos = _.shuffle(selectedUtxos)
+ }
+ selectedUtxos.reduce((total, utxo) => {
+ utxo.selected = total < payedAmount
+ total += utxo.amount
+ return total
+ }, 0)
+ },
+
+ //################### MEMPOOL API ###################
+ getAddressTxsDelayed: async function (addrData) {
+ const {
+ bitcoin: {addresses: addressesAPI}
+ } = mempoolJS()
+
+ const fn = async () =>
+ addressesAPI.getAddressTxs({
+ address: addrData.address
+ })
+ const addressTxs = await retryWithDelay(fn)
+ return this.addressHistoryFromTxs(addrData, addressTxs)
+ },
+
+ refreshRecommendedFees: async function () {
+ const {
+ bitcoin: {fees: feesAPI}
+ } = mempoolJS()
+
+ const fn = async () => feesAPI.getFeesRecommended()
+ this.payment.recommededFees = await retryWithDelay(fn)
+ },
+ getAddressTxsUtxoDelayed: async function (address) {
+ const {
+ bitcoin: {addresses: addressesAPI}
+ } = mempoolJS()
+
+ const fn = async () =>
+ addressesAPI.getAddressTxsUtxo({
+ address
+ })
+ return retryWithDelay(fn)
+ },
+ fetchTxHex: async function (txId) {
+ const {
+ bitcoin: {transactions: transactionsAPI}
+ } = mempoolJS()
+
+ try {
+ const response = await transactionsAPI.getTxHex({txid: txId})
+ return response
+ } catch (error) {
+ this.$q.notify({
+ type: 'warning',
+ message: `Failed to fetch transaction details for tx id: '${txId}'`,
+ timeout: 10000
+ })
+ LNbits.utils.notifyApiError(error)
+ throw error
+ }
+ },
+
+ //################### OTHER ###################
+ closeFormDialog: function () {
+ this.formDialog.data = {
+ is_unique: false
+ }
+ },
+ openQrCodeDialog: function (addressData) {
+ this.currentAddress = addressData
+ this.addresses.note = addressData.note || ''
+ this.addresses.show = true
+ },
+ searchInTab: function (tab, value) {
+ this.tab = tab
+ this[`${tab}Table`].filter = value
+ },
+
+ satBtc(val, showUnit = true) {
+ const value = this.config.data.sats_denominated
+ ? LNbits.utils.formatSat(val)
+ : val == 0
+ ? 0.0
+ : (val / 100000000).toFixed(8)
+ if (!showUnit) return value
+ return this.config.data.sats_denominated ? value + ' sat' : value + ' BTC'
+ },
+ getAccountDescription: function (accountType) {
+ return getAccountDescription(accountType)
+ }
+ },
+ created: async function () {
+ if (this.g.user.wallets.length) {
+ await this.getConfig()
+ await this.refreshWalletAccounts()
+ await this.refreshAddresses()
+ await this.scanAddressWithAmount()
+ }
+ }
+})
diff --git a/lnbits/extensions/watchonly/static/js/map.js b/lnbits/extensions/watchonly/static/js/map.js
new file mode 100644
index 000000000..d1bc80389
--- /dev/null
+++ b/lnbits/extensions/watchonly/static/js/map.js
@@ -0,0 +1,80 @@
+const mapAddressesData = a => ({
+ id: a.id,
+ address: a.address,
+ amount: a.amount,
+ wallet: a.wallet,
+ note: a.note,
+
+ isChange: a.branch_index === 1,
+ addressIndex: a.address_index,
+ hasActivity: a.has_activity
+})
+
+const mapInputToSentHistory = (tx, addressData, vin) => ({
+ sent: true,
+ txId: tx.txid,
+ address: addressData.address,
+ isChange: addressData.isChange,
+ amount: vin.prevout.value,
+ date: blockTimeToDate(tx.status.block_time),
+ height: tx.status.block_height,
+ confirmed: tx.status.confirmed,
+ fee: tx.fee,
+ expanded: false
+})
+
+const mapOutputToReceiveHistory = (tx, addressData, vout) => ({
+ received: true,
+ txId: tx.txid,
+ address: addressData.address,
+ isChange: addressData.isChange,
+ amount: vout.value,
+ date: blockTimeToDate(tx.status.block_time),
+ height: tx.status.block_height,
+ confirmed: tx.status.confirmed,
+ fee: tx.fee,
+ expanded: false
+})
+
+const mapUtxoToPsbtInput = utxo => ({
+ tx_id: utxo.txId,
+ vout: utxo.vout,
+ amount: utxo.amount,
+ address: utxo.address,
+ branch_index: utxo.isChange ? 1 : 0,
+ address_index: utxo.addressIndex,
+ masterpub_fingerprint: utxo.masterpubFingerprint,
+ accountType: utxo.accountType,
+ txHex: ''
+})
+
+const mapAddressDataToUtxo = (wallet, addressData, utxo) => ({
+ id: addressData.id,
+ address: addressData.address,
+ isChange: addressData.isChange,
+ addressIndex: addressData.addressIndex,
+ wallet: addressData.wallet,
+ accountType: addressData.accountType,
+ masterpubFingerprint: wallet.fingerprint,
+ txId: utxo.txid,
+ vout: utxo.vout,
+ confirmed: utxo.status.confirmed,
+ amount: utxo.value,
+ date: blockTimeToDate(utxo.status?.block_time),
+ sort: utxo.status?.block_time,
+ expanded: false,
+ selected: false
+})
+
+const mapWalletAccount = function (obj) {
+ obj._data = _.clone(obj)
+ obj.date = obj.time
+ ? Quasar.utils.date.formatDate(
+ new Date(obj.time * 1000),
+ 'YYYY-MM-DD HH:mm'
+ )
+ : ''
+ obj.label = obj.title // for drop-downs
+ obj.expanded = false
+ return obj
+}
diff --git a/lnbits/extensions/watchonly/static/js/tables.js b/lnbits/extensions/watchonly/static/js/tables.js
new file mode 100644
index 000000000..fdd558bde
--- /dev/null
+++ b/lnbits/extensions/watchonly/static/js/tables.js
@@ -0,0 +1,277 @@
+const tables = {
+ walletsTable: {
+ columns: [
+ {
+ name: 'new',
+ align: 'left',
+ label: ''
+ },
+ {
+ name: 'title',
+ align: 'left',
+ label: 'Title',
+ field: 'title'
+ },
+ {
+ name: 'amount',
+ align: 'left',
+ label: 'Amount'
+ },
+ {
+ name: 'type',
+ align: 'left',
+ label: 'Type',
+ field: 'type'
+ },
+ {name: 'id', align: 'left', label: 'ID', field: 'id'}
+ ],
+ pagination: {
+ rowsPerPage: 10
+ },
+ filter: ''
+ },
+ utxosTable: {
+ columns: [
+ {
+ name: 'expand',
+ align: 'left',
+ label: ''
+ },
+ {
+ name: 'selected',
+ align: 'left',
+ label: ''
+ },
+ {
+ name: 'status',
+ align: 'center',
+ label: 'Status',
+ sortable: true
+ },
+ {
+ name: 'address',
+ align: 'left',
+ label: 'Address',
+ field: 'address',
+ sortable: true
+ },
+ {
+ name: 'amount',
+ align: 'left',
+ label: 'Amount',
+ field: 'amount',
+ sortable: true
+ },
+ {
+ name: 'date',
+ align: 'left',
+ label: 'Date',
+ field: 'date',
+ sortable: true
+ },
+ {
+ name: 'wallet',
+ align: 'left',
+ label: 'Account',
+ field: 'wallet',
+ sortable: true
+ }
+ ],
+ pagination: {
+ rowsPerPage: 10
+ },
+ filter: ''
+ },
+ paymentTable: {
+ columns: [
+ {
+ name: 'data',
+ align: 'left'
+ }
+ ],
+ pagination: {
+ rowsPerPage: 10
+ },
+ filter: ''
+ },
+ summaryTable: {
+ columns: [
+ {
+ name: 'totalInputs',
+ align: 'center',
+ label: 'Selected Amount'
+ },
+ {
+ name: 'totalOutputs',
+ align: 'center',
+ label: 'Payed Amount'
+ },
+ {
+ name: 'fees',
+ align: 'center',
+ label: 'Fees'
+ },
+ {
+ name: 'change',
+ align: 'center',
+ label: 'Change'
+ }
+ ]
+ },
+ addressesTable: {
+ columns: [
+ {
+ name: 'expand',
+ align: 'left',
+ label: ''
+ },
+ {
+ name: 'address',
+ align: 'left',
+ label: 'Address',
+ field: 'address',
+ sortable: true
+ },
+ {
+ name: 'amount',
+ align: 'left',
+ label: 'Amount',
+ field: 'amount',
+ sortable: true
+ },
+ {
+ name: 'note',
+ align: 'left',
+ label: 'Note',
+ field: 'note',
+ sortable: true
+ },
+ {
+ name: 'wallet',
+ align: 'left',
+ label: 'Account',
+ field: 'wallet',
+ sortable: true
+ }
+ ],
+ pagination: {
+ rowsPerPage: 0,
+ sortBy: 'amount',
+ descending: true
+ },
+ filter: ''
+ },
+ historyTable: {
+ columns: [
+ {
+ name: 'expand',
+ align: 'left',
+ label: ''
+ },
+ {
+ name: 'status',
+ align: 'left',
+ label: 'Status'
+ },
+ {
+ name: 'amount',
+ align: 'left',
+ label: 'Amount',
+ field: 'amount',
+ sortable: true
+ },
+ {
+ name: 'address',
+ align: 'left',
+ label: 'Address',
+ field: 'address',
+ sortable: true
+ },
+ {
+ name: 'date',
+ align: 'left',
+ label: 'Date',
+ field: 'date',
+ sortable: true
+ }
+ ],
+ exportColums: [
+ {
+ label: 'Action',
+ field: 'action'
+ },
+ {
+ label: 'Date&Time',
+ field: 'date'
+ },
+ {
+ label: 'Amount',
+ field: 'amount'
+ },
+ {
+ label: 'Fee',
+ field: 'fee'
+ },
+ {
+ label: 'Transaction Id',
+ field: 'txId'
+ }
+ ],
+ pagination: {
+ rowsPerPage: 0
+ },
+ filter: ''
+ }
+}
+
+const tableData = {
+ walletAccounts: [],
+ addresses: {
+ show: false,
+ data: [],
+ history: [],
+ selectedWallet: null,
+ note: '',
+ filterOptions: [
+ 'Show Change Addresses',
+ 'Show Gap Addresses',
+ 'Only With Amount'
+ ],
+ filterValues: []
+ },
+ utxos: {
+ data: [],
+ total: 0
+ },
+ payment: {
+ data: [{address: '', amount: undefined}],
+ changeWallet: null,
+ changeAddress: {},
+ changeAmount: 0,
+
+ feeRate: 1,
+ recommededFees: {
+ fastestFee: 1,
+ halfHourFee: 1,
+ hourFee: 1,
+ economyFee: 1,
+ minimumFee: 1
+ },
+ fee: 0,
+ txSize: 0,
+ psbtBase64: '',
+ utxoSelectionModes: [
+ 'Manual',
+ 'Random',
+ 'Select All',
+ 'Smaller Inputs First',
+ 'Larger Inputs First'
+ ],
+ utxoSelectionMode: 'Manual',
+ show: false,
+ showAdvanced: false
+ },
+ summary: {
+ data: [{totalInputs: 0, totalOutputs: 0, fees: 0, change: 0}]
+ }
+}
diff --git a/lnbits/extensions/watchonly/static/js/utils.js b/lnbits/extensions/watchonly/static/js/utils.js
new file mode 100644
index 000000000..26bebac66
--- /dev/null
+++ b/lnbits/extensions/watchonly/static/js/utils.js
@@ -0,0 +1,99 @@
+const blockTimeToDate = blockTime =>
+ blockTime ? moment(blockTime * 1000).format('LLL') : ''
+
+const currentDateTime = () => moment().format('LLL')
+
+const sleep = ms => new Promise(r => setTimeout(r, ms))
+
+const retryWithDelay = async function (fn, retryCount = 0) {
+ try {
+ await sleep(25)
+ // Do not return the call directly, use result.
+ // Otherwise the error will not be cought in this try-catch block.
+ const result = await fn()
+ return result
+ } catch (err) {
+ if (retryCount > 100) throw err
+ await sleep((retryCount + 1) * 1000)
+ return retryWithDelay(fn, retryCount + 1)
+ }
+}
+
+const txSize = tx => {
+ // https://bitcoinops.org/en/tools/calc-size/
+ // overhead size
+ const nVersion = 4
+ const inCount = 1
+ const outCount = 1
+ const nlockTime = 4
+ const hasSegwit = !!tx.inputs.find(inp =>
+ ['p2wsh', 'p2wpkh', 'p2tr'].includes(inp.accountType)
+ )
+ const segwitFlag = hasSegwit ? 0.5 : 0
+ const overheadSize = nVersion + inCount + outCount + nlockTime + segwitFlag
+
+ // inputs size
+ const outpoint = 36 // txId plus vout index number
+ const scriptSigLength = 1
+ const nSequence = 4
+ const inputsSize = tx.inputs.reduce((t, inp) => {
+ const scriptSig =
+ inp.accountType === 'p2pkh' ? 107 : inp.accountType === 'p2sh' ? 254 : 0
+ const witnessItemCount = hasSegwit ? 0.25 : 0
+ const witnessItems =
+ inp.accountType === 'p2wpkh'
+ ? 27
+ : inp.accountType === 'p2wsh'
+ ? 63.5
+ : inp.accountType === 'p2tr'
+ ? 16.5
+ : 0
+ t +=
+ outpoint +
+ scriptSigLength +
+ nSequence +
+ scriptSig +
+ witnessItemCount +
+ witnessItems
+ return t
+ }, 0)
+
+ // outputs size
+ const nValue = 8
+ const scriptPubKeyLength = 1
+
+ const outputsSize = tx.outputs.reduce((t, out) => {
+ const type = guessAddressType(out.address)
+
+ const scriptPubKey =
+ type === 'p2pkh'
+ ? 25
+ : type === 'p2wpkh'
+ ? 22
+ : type === 'p2sh'
+ ? 23
+ : type === 'p2wsh'
+ ? 34
+ : 34 // default to the largest size (p2tr included)
+ t += nValue + scriptPubKeyLength + scriptPubKey
+ return t
+ }, 0)
+
+ return overheadSize + inputsSize + outputsSize
+}
+const guessAddressType = (a = '') => {
+ if (a.startsWith('1') || a.startsWith('n')) return 'p2pkh'
+ if (a.startsWith('3') || a.startsWith('2')) return 'p2sh'
+ if (a.startsWith('bc1q') || a.startsWith('tb1q'))
+ return a.length === 42 ? 'p2wpkh' : 'p2wsh'
+ if (a.startsWith('bc1p') || a.startsWith('tb1p')) return 'p2tr'
+}
+
+const ACCOUNT_TYPES = {
+ p2tr: 'Taproot, BIP86, P2TR, Bech32m',
+ p2wpkh: 'SegWit, BIP84, P2WPKH, Bech32',
+ p2sh: 'BIP49, P2SH-P2WPKH, Base58',
+ p2pkh: 'Legacy, BIP44, P2PKH, Base58'
+}
+
+const getAccountDescription = type => ACCOUNT_TYPES[type] || 'nonstandard'
diff --git a/lnbits/extensions/watchonly/templates/watchonly/_api_docs.html b/lnbits/extensions/watchonly/templates/watchonly/_api_docs.html
index 94b44a443..db0811f57 100644
--- a/lnbits/extensions/watchonly/templates/watchonly/_api_docs.html
+++ b/lnbits/extensions/watchonly/templates/watchonly/_api_docs.html
@@ -1,248 +1,27 @@
- Watch Only extension uses mempool.space
+ Onchain Wallet (watch-only) extension uses mempool.space
For use with "account Extended Public Key"
https://iancoleman.io/bip39/
Created by,
- Ben Arc (using,
- Ben Arc
+ (using,
+ Embit)
+
+
+ Swagger REST API Documentation
-
-
-
-
-
-
- GET /watchonly/api/v1/wallet
- Headers
- {"X-Api-Key": <invoice_key>}
-
- Body (application/json)
-
-
- Returns 200 OK (application/json)
-
- [<wallets_object>, ...]
- Curl example
- curl -X GET {{ request.base_url }}watchonly/api/v1/wallet -H
- "X-Api-Key: {{ user.wallets[0].inkey }}"
-
-
-
-
-
-
-
- GET
- /watchonly/api/v1/wallet/<wallet_id>
- Headers
- {"X-Api-Key": <invoice_key>}
-
- Body (application/json)
-
-
- Returns 201 CREATED (application/json)
-
- [<wallet_object>, ...]
- Curl example
- curl -X GET {{ request.base_url
- }}watchonly/api/v1/wallet/<wallet_id> -H "X-Api-Key: {{
- user.wallets[0].inkey }}"
-
-
-
-
-
-
-
- POST /watchonly/api/v1/wallet
- Headers
- {"X-Api-Key": <admin_key>}
-
- Body (application/json)
-
-
- Returns 201 CREATED (application/json)
-
- [<wallet_object>, ...]
- Curl example
- curl -X POST {{ request.base_url }}watchonly/api/v1/wallet -d
- '{"title": <string>, "masterpub": <string>}' -H
- "Content-type: application/json" -H "X-Api-Key: {{
- user.wallets[0].adminkey }}"
-
-
-
-
-
-
-
- DELETE
- /watchonly/api/v1/wallet/<wallet_id>
- Headers
- {"X-Api-Key": <admin_key>}
- Returns 204 NO CONTENT
-
- Curl example
- curl -X DELETE {{ request.base_url
- }}watchonly/api/v1/wallet/<wallet_id> -H "X-Api-Key: {{
- user.wallets[0].adminkey }}"
-
-
-
-
-
-
-
-
- GET
- /watchonly/api/v1/addresses/<wallet_id>
- Headers
- {"X-Api-Key": <invoice_key>}
-
- Body (application/json)
-
-
- Returns 200 OK (application/json)
-
- [<address_object>, ...]
- Curl example
- curl -X GET {{ request.base_url
- }}watchonly/api/v1/addresses/<wallet_id> -H "X-Api-Key: {{
- user.wallets[0].inkey }}"
-
-
-
-
-
-
-
-
- GET
- /watchonly/api/v1/address/<wallet_id>
- Headers
- {"X-Api-Key": <invoice_key>}
-
- Body (application/json)
-
-
- Returns 200 OK (application/json)
-
- [<address_object>, ...]
- Curl example
- curl -X GET {{ request.base_url
- }}watchonly/api/v1/address/<wallet_id> -H "X-Api-Key: {{
- user.wallets[0].inkey }}"
-
-
-
-
-
-
-
-
- GET /watchonly/api/v1/mempool
- Headers
- {"X-Api-Key": <admin_key>}
-
- Body (application/json)
-
-
- Returns 200 OK (application/json)
-
- [<mempool_object>, ...]
- Curl example
- curl -X GET {{ request.base_url }}watchonly/api/v1/mempool -H
- "X-Api-Key: {{ user.wallets[0].adminkey }}"
-
-
-
-
-
-
-
-
- POST
- /watchonly/api/v1/mempool
- Headers
- {"X-Api-Key": <admin_key>}
-
- Body (application/json)
-
-
- Returns 201 CREATED (application/json)
-
- [<mempool_object>, ...]
- Curl example
- curl -X PUT {{ request.base_url }}watchonly/api/v1/mempool -d
- '{"endpoint": <string>}' -H "Content-type: application/json"
- -H "X-Api-Key: {{ user.wallets[0].adminkey }}"
-
-
-
-
-
diff --git a/lnbits/extensions/watchonly/templates/watchonly/index.html b/lnbits/extensions/watchonly/templates/watchonly/index.html
index e70f8a23c..0ab2a67be 100644
--- a/lnbits/extensions/watchonly/templates/watchonly/index.html
+++ b/lnbits/extensions/watchonly/templates/watchonly/index.html
@@ -3,36 +3,36 @@
-
- {% raw %}
- New wallet
-
-
-
- Point to another Mempool
- {{ this.mempool.endpoint }}
-
-
-
- set
- cancel
-
-
+ {% raw %}
+
+
+
+ {{satBtc(utxos.total)}}
-
-
+
+
+
+
+
+
- Wallets
+ Add Wallet Account
+
-
+
+
+
@@ -73,31 +73,97 @@
- Adresses
-
-
+ @click="props.row.expanded= !props.row.expanded"
+ :icon="props.row.expanded? 'remove' : 'add'"
+ />
-
- {{ col.value }}
+
+
+ New Receive Address
+
+
+
+
+ {{props.row.title}}
+
+
+ {{getAmmountForWallet(props.row.id)}}
+
+
+ {{props.row.type}}
+
+
+ {{props.row.id}}
+
+
+
+
+
+
+
+ New Receive Address
+
+
+ {{getAccountDescription(props.row.type)}}
+
+
+
+
+
+ Master Pubkey:
+
+
+
+
+
+
+ Last Address Index:
+
+ {{props.row.address_no}}
+ none
+
+
+
+
+ Fingerprint:
+ {{props.row.fingerprint}}
+
+
+
+
+
+ Delete
+
+
+
+
@@ -106,70 +172,862 @@
-
- {{satBtc(utxos.total)}}
-
- {{utxos.sats ? ' sats' : ' BTC'}}
+
+
+ Scan Blockchain
+
+
+
+ Make Payment
+
+
+
+
+
-
-
- Transactions
-
-
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
-
-
+
+
+
+
+
+
+
+
+
+
+
+ {{satBtc(props.row.amount)}}
+
+
+
+ {{props.row.note}}
+
+
+ {{getWalletName(props.row.wallet)}}
+
+
+
+
+
+
+
+ QR Code
+
+
+ Rescan
+
+
+ History
+
+
+ View Coins
+
+
+
+
+ Note:
+
+
+
+
+ Update
+
+
+
+
+
+
+ {{props.row.error}}
+
+
+
+
+
+ Gap limit of 20 addresses exceeded. Other wallets
+ might not detect funds at this address.
+
+
+
+
-
-
-
-
-
-
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Export to CSV
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{props.row.confirmed ? 'Sent' : 'Sending...'}}
+
+
+ {{props.row.confirmed ? 'Received' : 'Receiving...'}}
+
+
+
+
+ {{satBtc(props.row.totalAmount || props.row.amount)}}
+
+
+
+
+ {{props.row.address}}
+
+ ...
+
+
+ {{ props.row.date }}
+
+
+
+
+ Transaction Id
+
+
+
+ UTXOs
+
+ {{satBtc(props.row.amount)}}
+
+ {{props.row.address}}
+
+
+
+ {{satBtc(s.amount)}}
+ {{s.address}}
+
+
+ Fee
+ {{satBtc(props.row.fee)}}
+
+
+ Block Height
+ {{props.row.height}}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Max
+
+
+
+
+
+
+
+
+
+ Add Send Address
+
+
+
+
+ Payed Amount:
+
+
+ {{satBtc(getTotalPaymentAmount())}}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Confirmed
+
+
+ Pending
+
+
+
+
+
+
+ {{props.row.address}}
+
+ change
+
+
+
+
+
+ {{satBtc(props.row.amount)}}
+
+
+
+ {{ props.row.date }}
+
+
+ {{getWalletName(props.row.wallet)}}
+
+
+
+
+
+ Transaction Id
+
+
+
+
+
+
+
+
+
+
+
+
+ Slected Amount:
+
+
+ {{satBtc(getTotalSelectedUtxoAmount())}}
+
+
+
+
+
+
+
+
+
- {{ col.label }}
-
-
-
-
-
-
-
+
+
+
+
+
+
+ Change Account:
+
+
+
+
+
+
+
+
+ Fee Rate:
+
+
+
+
+
+
+
+
+
+
+
+ Warning! The fee is too low. The transaction might take
+ a long time to confirm.
+
+
+ Warning! The fee is too high. You might be overpaying
+ for this transaction.
+
+
+
+
+
+ Fee:
+ {{computeFee()}} sats
+
+ Refresh Fee Rates
+
+
+
- {{ col.name == 'value' ? satBtc(col.value) : col.value }}
-
-
-
-
+
+
+
+
+
+
+
+
+ {{satBtc(getTotalSelectedUtxoAmount())}}
+
+
+
+
+ {{satBtc(getTotalPaymentAmount())}}
+
+
+
+
+ {{satBtc(computeFee())}}
+
+
+
+
+ {{payment.changeAmount ? payment.changeAmount:
+ 'no change'}}
+
+
+ Below dust limit. Will be used as feee.
+
+
+
+
+
+
+
+
+
+ Create PSBT
+
+
+
+ The payed amount is higher than the selected amount!
+
+
+
+
+
+
+
+
+
+
+
+
+
@@ -180,7 +1038,7 @@
- {{SITE_TITLE}} Watch Only Extension
+ {{SITE_TITLE}} Onchain Wallet (watch-only) Extension
@@ -192,7 +1050,7 @@
-
+
Create Watch-only WalletAdd Watch-Only Account
+ Cancel
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Update
Cancel
-
-
+
+
{% raw %}
- Addresses
+
+ Address Details
-
- Current:
- {{ currentaddress }}
-
-
+
-
-
-
-
- {{ data.address }}
-
-
-
-
+
+ {{ currentAddress.address }}
+
-
+
+
+
+
+ Gap limit of 20 addresses exceeded. Other wallets might not detect
+ funds at this address.
+
Get fresh address Save Note
Close
+
{% endraw %}
+
{% endblock %} {% block scripts %} {{ window_vars(user) }}
-
-
+
+
+
+
{% endblock %}
diff --git a/lnbits/extensions/watchonly/views_api.py b/lnbits/extensions/watchonly/views_api.py
index 0cca67bc4..f9055a207 100644
--- a/lnbits/extensions/watchonly/views_api.py
+++ b/lnbits/extensions/watchonly/views_api.py
@@ -1,6 +1,11 @@
from http import HTTPStatus
-from fastapi import Query
+from embit import script
+from embit.descriptor import Descriptor, Key
+from embit.ec import PublicKey
+from embit.psbt import PSBT, DerivationPath
+from embit.transaction import Transaction, TransactionInput, TransactionOutput
+from fastapi import Query, Request
from fastapi.params import Depends
from starlette.exceptions import HTTPException
@@ -8,17 +13,25 @@ from lnbits.decorators import WalletTypeInfo, get_key_type, require_admin_key
from lnbits.extensions.watchonly import watchonly_ext
from .crud import (
+ create_config,
+ create_fresh_addresses,
create_mempool,
create_watch_wallet,
+ delete_addresses_for_wallet,
delete_watch_wallet,
get_addresses,
+ get_config,
get_fresh_address,
get_mempool,
get_watch_wallet,
get_watch_wallets,
+ update_address,
+ update_config,
update_mempool,
+ update_watch_wallet,
)
-from .models import CreateWallet
+from .helpers import parse_key
+from .models import Config, CreatePsbt, CreateWallet, WalletAccount
###################WALLETS#############################
@@ -48,18 +61,42 @@ async def api_wallet_retrieve(
@watchonly_ext.post("/api/v1/wallet")
async def api_wallet_create_or_update(
- data: CreateWallet, wallet_id=None, w: WalletTypeInfo = Depends(require_admin_key)
+ data: CreateWallet, w: WalletTypeInfo = Depends(require_admin_key)
):
try:
- wallet = await create_watch_wallet(
- user=w.wallet.user, masterpub=data.masterpub, title=data.title
+ (descriptor, _) = parse_key(data.masterpub)
+
+ new_wallet = WalletAccount(
+ id="none",
+ user=w.wallet.user,
+ masterpub=data.masterpub,
+ fingerprint=descriptor.keys[0].fingerprint.hex(),
+ type=descriptor.scriptpubkey_type(),
+ title=data.title,
+ address_no=-1, # so fresh address on empty wallet can get address with index 0
+ balance=0,
)
+
+ wallets = await get_watch_wallets(w.wallet.user)
+ existing_wallet = next(
+ (ew for ew in wallets if ew.fingerprint == new_wallet.fingerprint), None
+ )
+ if existing_wallet:
+ raise ValueError(
+ "Account '{}' has the same master pulic key".format(
+ existing_wallet.title
+ )
+ )
+
+ wallet = await create_watch_wallet(new_wallet)
+
+ await api_get_addresses(wallet.id, w)
except Exception as e:
raise HTTPException(status_code=HTTPStatus.BAD_REQUEST, detail=str(e))
- mempool = await get_mempool(w.wallet.user)
- if not mempool:
- create_mempool(user=w.wallet.user)
+ config = await get_config(w.wallet.user)
+ if not config:
+ await create_config(user=w.wallet.user)
return wallet.dict()
@@ -73,6 +110,7 @@ async def api_wallet_delete(wallet_id, w: WalletTypeInfo = Depends(require_admin
)
await delete_watch_wallet(wallet_id)
+ await delete_addresses_for_wallet(wallet_id)
raise HTTPException(status_code=HTTPStatus.NO_CONTENT)
@@ -83,31 +121,171 @@ async def api_wallet_delete(wallet_id, w: WalletTypeInfo = Depends(require_admin
@watchonly_ext.get("/api/v1/address/{wallet_id}")
async def api_fresh_address(wallet_id, w: WalletTypeInfo = Depends(get_key_type)):
address = await get_fresh_address(wallet_id)
+ return address.dict()
- return [address.dict()]
+
+@watchonly_ext.put("/api/v1/address/{id}")
+async def api_update_address(
+ id: str, req: Request, w: WalletTypeInfo = Depends(require_admin_key)
+):
+ body = await req.json()
+ params = {}
+ # amout is only updated if the address has history
+ if "amount" in body:
+ params["amount"] = int(body["amount"])
+ params["has_activity"] = True
+
+ if "note" in body:
+ params["note"] = str(body["note"])
+
+ address = await update_address(**params, id=id)
+
+ wallet = (
+ await get_watch_wallet(address.wallet)
+ if address.branch_index == 0 and address.amount != 0
+ else None
+ )
+
+ if wallet and wallet.address_no < address.address_index:
+ await update_watch_wallet(
+ address.wallet, **{"address_no": address.address_index}
+ )
+ return address
@watchonly_ext.get("/api/v1/addresses/{wallet_id}")
async def api_get_addresses(wallet_id, w: WalletTypeInfo = Depends(get_key_type)):
wallet = await get_watch_wallet(wallet_id)
-
if not wallet:
raise HTTPException(
status_code=HTTPStatus.NOT_FOUND, detail="Wallet does not exist."
)
addresses = await get_addresses(wallet_id)
+ config = await get_config(w.wallet.user)
if not addresses:
- await get_fresh_address(wallet_id)
+ await create_fresh_addresses(wallet_id, 0, config.receive_gap_limit)
+ await create_fresh_addresses(wallet_id, 0, config.change_gap_limit, True)
addresses = await get_addresses(wallet_id)
+ receive_addresses = list(filter(lambda addr: addr.branch_index == 0, addresses))
+ change_addresses = list(filter(lambda addr: addr.branch_index == 1, addresses))
+
+ last_receive_address = list(
+ filter(lambda addr: addr.has_activity, receive_addresses)
+ )[-1:]
+ last_change_address = list(
+ filter(lambda addr: addr.has_activity, change_addresses)
+ )[-1:]
+
+ if last_receive_address:
+ current_index = receive_addresses[-1].address_index
+ address_index = last_receive_address[0].address_index
+ await create_fresh_addresses(
+ wallet_id, current_index + 1, address_index + config.receive_gap_limit + 1
+ )
+
+ if last_change_address:
+ current_index = change_addresses[-1].address_index
+ address_index = last_change_address[0].address_index
+ await create_fresh_addresses(
+ wallet_id,
+ current_index + 1,
+ address_index + config.change_gap_limit + 1,
+ True,
+ )
+
+ addresses = await get_addresses(wallet_id)
return [address.dict() for address in addresses]
+#############################PSBT##########################
+
+
+@watchonly_ext.post("/api/v1/psbt")
+async def api_psbt_create(
+ data: CreatePsbt, w: WalletTypeInfo = Depends(require_admin_key)
+):
+ try:
+ vin = [
+ TransactionInput(bytes.fromhex(inp.tx_id), inp.vout) for inp in data.inputs
+ ]
+ vout = [
+ TransactionOutput(out.amount, script.address_to_scriptpubkey(out.address))
+ for out in data.outputs
+ ]
+
+ descriptors = {}
+ for _, masterpub in enumerate(data.masterpubs):
+ descriptors[masterpub.fingerprint] = parse_key(masterpub.public_key)
+
+ inputs_extra = []
+ bip32_derivations = {}
+ for i, inp in enumerate(data.inputs):
+ descriptor = descriptors[inp.masterpub_fingerprint][0]
+ d = descriptor.derive(inp.address_index, inp.branch_index)
+ for k in d.keys:
+ bip32_derivations[PublicKey.parse(k.sec())] = DerivationPath(
+ k.origin.fingerprint, k.origin.derivation
+ )
+ inputs_extra.append(
+ {
+ "bip32_derivations": bip32_derivations,
+ "non_witness_utxo": Transaction.from_string(inp.tx_hex),
+ }
+ )
+
+ tx = Transaction(vin=vin, vout=vout)
+ psbt = PSBT(tx)
+
+ for i, inp in enumerate(inputs_extra):
+ psbt.inputs[i].bip32_derivations = inp["bip32_derivations"]
+ psbt.inputs[i].non_witness_utxo = inp.get("non_witness_utxo", None)
+
+ outputs_extra = []
+ bip32_derivations = {}
+ for i, out in enumerate(data.outputs):
+ if out.branch_index == 1:
+ descriptor = descriptors[out.masterpub_fingerprint][0]
+ d = descriptor.derive(out.address_index, out.branch_index)
+ for k in d.keys:
+ bip32_derivations[PublicKey.parse(k.sec())] = DerivationPath(
+ k.origin.fingerprint, k.origin.derivation
+ )
+ outputs_extra.append({"bip32_derivations": bip32_derivations})
+
+ for i, out in enumerate(outputs_extra):
+ psbt.outputs[i].bip32_derivations = out["bip32_derivations"]
+
+ return psbt.to_string()
+
+ except Exception as e:
+ raise HTTPException(status_code=HTTPStatus.BAD_REQUEST, detail=str(e))
+
+
+#############################CONFIG##########################
+
+
+@watchonly_ext.put("/api/v1/config")
+async def api_update_config(
+ data: Config, w: WalletTypeInfo = Depends(require_admin_key)
+):
+ config = await update_config(data, user=w.wallet.user)
+ return config.dict()
+
+
+@watchonly_ext.get("/api/v1/config")
+async def api_get_config(w: WalletTypeInfo = Depends(get_key_type)):
+ config = await get_config(w.wallet.user)
+ if not config:
+ config = await create_config(user=w.wallet.user)
+ return config.dict()
+
+
#############################MEMPOOL##########################
-
+### TODO: fix statspay dependcy and remove
@watchonly_ext.put("/api/v1/mempool")
async def api_update_mempool(
endpoint: str = Query(...), w: WalletTypeInfo = Depends(require_admin_key)
@@ -116,6 +294,7 @@ async def api_update_mempool(
return mempool.dict()
+### TODO: fix statspay dependcy and remove
@watchonly_ext.get("/api/v1/mempool")
async def api_get_mempool(w: WalletTypeInfo = Depends(require_admin_key)):
mempool = await get_mempool(w.wallet.user)
diff --git a/lnbits/helpers.py b/lnbits/helpers.py
index 71b3dd691..e97fc7bbb 100644
--- a/lnbits/helpers.py
+++ b/lnbits/helpers.py
@@ -34,7 +34,7 @@ class ExtensionManager:
@property
def extensions(self) -> List[Extension]:
- output = []
+ output: List[Extension] = []
if "all" in self._disabled:
return output
diff --git a/lnbits/jinja2_templating.py b/lnbits/jinja2_templating.py
index 5abcd0bff..385703d19 100644
--- a/lnbits/jinja2_templating.py
+++ b/lnbits/jinja2_templating.py
@@ -21,7 +21,7 @@ class Jinja2Templates(templating.Jinja2Templates):
self.env = self.get_environment(loader)
def get_environment(self, loader: "jinja2.BaseLoader") -> "jinja2.Environment":
- @jinja2.contextfunction
+ @jinja2.pass_context
def url_for(context: dict, name: str, **path_params: typing.Any) -> str:
request: Request = context["request"]
return request.app.url_path_for(name, **path_params)
diff --git a/lnbits/static/js/base.js b/lnbits/static/js/base.js
index cd5fd1c4c..579db400b 100644
--- a/lnbits/static/js/base.js
+++ b/lnbits/static/js/base.js
@@ -261,7 +261,7 @@ window.LNbits = {
return data
}
},
- exportCSV: function (columns, data) {
+ exportCSV: function (columns, data, fileName) {
var wrapCsvValue = function (val, formatFn) {
var formatted = formatFn !== void 0 ? formatFn(val) : val
@@ -295,7 +295,7 @@ window.LNbits = {
.join('\r\n')
var status = Quasar.utils.exportFile(
- 'table-export.csv',
+ `${fileName || 'table-export'}.csv`,
content,
'text/csv'
)
diff --git a/lnbits/tasks.py b/lnbits/tasks.py
index 86863f98f..f4d0a928d 100644
--- a/lnbits/tasks.py
+++ b/lnbits/tasks.py
@@ -66,7 +66,7 @@ async def webhook_handler():
raise HTTPException(status_code=HTTPStatus.NO_CONTENT)
-internal_invoice_queue = asyncio.Queue(0)
+internal_invoice_queue: asyncio.Queue = asyncio.Queue(0)
async def internal_invoice_listener():
diff --git a/lnbits/utils/exchange_rates.py b/lnbits/utils/exchange_rates.py
index 0432b364a..2801146b5 100644
--- a/lnbits/utils/exchange_rates.py
+++ b/lnbits/utils/exchange_rates.py
@@ -1,5 +1,5 @@
import asyncio
-from typing import Callable, NamedTuple
+from typing import Callable, List, NamedTuple
import httpx
from loguru import logger
@@ -227,10 +227,10 @@ async def btc_price(currency: str) -> float:
"TO": currency.upper(),
"to": currency.lower(),
}
- rates = []
- tasks = []
+ rates: List[float] = []
+ tasks: List[asyncio.Task] = []
- send_channel = asyncio.Queue()
+ send_channel: asyncio.Queue = asyncio.Queue()
async def controller():
failures = 0
diff --git a/lnbits/wallets/eclair.py b/lnbits/wallets/eclair.py
index c878d0d19..ab99c699d 100644
--- a/lnbits/wallets/eclair.py
+++ b/lnbits/wallets/eclair.py
@@ -7,7 +7,10 @@ from typing import AsyncGenerator, Dict, Optional
import httpx
from loguru import logger
-from websockets import connect
+
+# TODO: https://github.com/lnbits/lnbits-legend/issues/764
+# mypy https://github.com/aaugustin/websockets/issues/940
+from websockets import connect # type: ignore
from websockets.exceptions import (
ConnectionClosed,
ConnectionClosedError,
diff --git a/lnbits/wallets/fake.py b/lnbits/wallets/fake.py
index 3859d33c5..3126ee46a 100644
--- a/lnbits/wallets/fake.py
+++ b/lnbits/wallets/fake.py
@@ -28,7 +28,7 @@ class FakeWallet(Wallet):
logger.info(
"FakeWallet funding source is for using LNbits as a centralised, stand-alone payment system with brrrrrr."
)
- return StatusResponse(None, float("inf"))
+ return StatusResponse(None, 1000000000)
async def create_invoice(
self,
@@ -82,7 +82,7 @@ class FakeWallet(Wallet):
invoice = decode(bolt11)
if (
hasattr(invoice, "checking_id")
- and invoice.checking_id[6:] == data["privkey"][:6]
+ and invoice.checking_id[6:] == data["privkey"][:6] # type: ignore
):
return PaymentResponse(True, invoice.payment_hash, 0)
else:
@@ -97,7 +97,7 @@ class FakeWallet(Wallet):
return PaymentStatus(None)
async def paid_invoices_stream(self) -> AsyncGenerator[str, None]:
- self.queue = asyncio.Queue(0)
+ self.queue: asyncio.Queue = asyncio.Queue(0)
while True:
value = await self.queue.get()
yield value
diff --git a/lnbits/wallets/lnpay.py b/lnbits/wallets/lnpay.py
index 7171ec3fb..18b4f8bbe 100644
--- a/lnbits/wallets/lnpay.py
+++ b/lnbits/wallets/lnpay.py
@@ -119,7 +119,7 @@ class LNPayWallet(Wallet):
return PaymentStatus(statuses[r.json()["settled"]])
async def paid_invoices_stream(self) -> AsyncGenerator[str, None]:
- self.queue = asyncio.Queue(0)
+ self.queue: asyncio.Queue = asyncio.Queue(0)
while True:
value = await self.queue.get()
yield value
diff --git a/lnbits/wallets/macaroon/macaroon.py b/lnbits/wallets/macaroon/macaroon.py
index 2183dacb8..091551238 100644
--- a/lnbits/wallets/macaroon/macaroon.py
+++ b/lnbits/wallets/macaroon/macaroon.py
@@ -73,10 +73,10 @@ class AESCipher(object):
final_key += key
return final_key[:output]
- def decrypt(self, encrypted: str) -> str:
+ def decrypt(self, encrypted: str) -> str: # type: ignore
"""Decrypts a string using AES-256-CBC."""
passphrase = self.passphrase
- encrypted = base64.b64decode(encrypted)
+ encrypted = base64.b64decode(encrypted) # type: ignore
assert encrypted[0:8] == b"Salted__"
salt = encrypted[8:16]
key_iv = self.bytes_to_key(passphrase.encode(), salt, 32 + 16)
@@ -84,7 +84,7 @@ class AESCipher(object):
iv = key_iv[32:]
aes = AES.new(key, AES.MODE_CBC, iv)
try:
- return self.unpad(aes.decrypt(encrypted[16:])).decode()
+ return self.unpad(aes.decrypt(encrypted[16:])).decode() # type: ignore
except UnicodeDecodeError:
raise ValueError("Wrong passphrase")
diff --git a/lnbits/wallets/opennode.py b/lnbits/wallets/opennode.py
index d503b4dd9..be82365dd 100644
--- a/lnbits/wallets/opennode.py
+++ b/lnbits/wallets/opennode.py
@@ -127,7 +127,7 @@ class OpenNodeWallet(Wallet):
return PaymentStatus(statuses[r.json()["data"]["status"]])
async def paid_invoices_stream(self) -> AsyncGenerator[str, None]:
- self.queue = asyncio.Queue(0)
+ self.queue: asyncio.Queue = asyncio.Queue(0)
while True:
value = await self.queue.get()
yield value
diff --git a/mypy.ini b/mypy.ini
index 735f89e05..e5a974b51 100644
--- a/mypy.ini
+++ b/mypy.ini
@@ -1,7 +1,8 @@
[mypy]
ignore_missing_imports = True
-exclude = lnbits/wallets/lnd_grpc_files/
-exclude = lnbits/extensions/
-
+exclude = (?x)(
+ ^lnbits/extensions.
+ | ^lnbits/wallets/lnd_grpc_files.
+ )
[mypy-lnbits.wallets.lnd_grpc_files.*]
follow_imports = skip
diff --git a/result b/result
new file mode 120000
index 000000000..b0acd55c0
--- /dev/null
+++ b/result
@@ -0,0 +1 @@
+/nix/store/ds9c48q7hnkdmpzy3aq14kc1x9wrrszd-python3.9-lnbits-0.1.0
\ No newline at end of file
diff --git a/tests/core/views/test_api.py b/tests/core/views/test_api.py
index 6a5f82ecb..dfd2b32ae 100644
--- a/tests/core/views/test_api.py
+++ b/tests/core/views/test_api.py
@@ -1,6 +1,7 @@
import pytest
import pytest_asyncio
from lnbits.core.crud import get_wallet
+from lnbits.core.views.api import api_payment
from ...helpers import get_random_invoice_data
@@ -155,3 +156,26 @@ async def test_decode_invoice(client, invoice):
)
assert response.status_code < 300
assert response.json()["payment_hash"] == invoice["payment_hash"]
+
+
+# check api_payment() internal function call (NOT API): payment status
+@pytest.mark.asyncio
+async def test_api_payment_without_key(invoice):
+ # check the payment status
+ response = await api_payment(invoice["payment_hash"])
+ assert type(response) == dict
+ assert response["paid"] == True
+ # no key, that's why no "details"
+ assert "details" not in response
+
+
+# check api_payment() internal function call (NOT API): payment status
+@pytest.mark.asyncio
+async def test_api_payment_with_key(invoice, inkey_headers_from):
+ # check the payment status
+ response = await api_payment(
+ invoice["payment_hash"], inkey_headers_from["X-Api-Key"]
+ )
+ assert type(response) == dict
+ assert response["paid"] == True
+ assert "details" in response
diff --git a/tests/data/mock_data.zip b/tests/data/mock_data.zip
index 0480adc27..6f7165b36 100644
Binary files a/tests/data/mock_data.zip and b/tests/data/mock_data.zip differ
diff --git a/tools/conv.py b/tools/conv.py
index 99bb54f02..b93bcfbea 100644
--- a/tools/conv.py
+++ b/tools/conv.py
@@ -1,9 +1,8 @@
-import psycopg2
-import sqlite3
-import os
import argparse
+import os
+import sqlite3
-
+import psycopg2
from environs import Env # type: ignore
env = Env()
@@ -284,22 +283,24 @@ def migrate_ext(sqlite_db_file, schema, ignore_missing=True):
masterpub,
title,
address_no,
- balance
+ balance,
+ type,
+ fingerprint
)
- VALUES (%s, %s, %s, %s, %s, %s);
+ VALUES (%s, %s, %s, %s, %s, %s, %s, %s);
"""
insert_to_pg(q, res.fetchall())
# ADDRESSES
res = sq.execute("SELECT * FROM addresses;")
q = f"""
- INSERT INTO watchonly.addresses (id, address, wallet, amount)
- VALUES (%s, %s, %s, %s);
+ INSERT INTO watchonly.addresses (id, address, wallet, amount, branch_index, address_index, has_activity, note)
+ VALUES (%s, %s, %s, %s, %s, %s, %s::boolean, %s);
"""
insert_to_pg(q, res.fetchall())
- # MEMPOOL
- res = sq.execute("SELECT * FROM mempool;")
+ # CONFIG
+ res = sq.execute("SELECT * FROM config;")
q = f"""
- INSERT INTO watchonly.mempool ("user", endpoint)
+ INSERT INTO watchonly.config ("user", json_data)
VALUES (%s, %s);
"""
insert_to_pg(q, res.fetchall())
@@ -540,8 +541,8 @@ def migrate_ext(sqlite_db_file, schema, ignore_missing=True):
# ITEMS
res = sq.execute("SELECT * FROM items;")
q = f"""
- INSERT INTO offlineshop.items (shop, id, name, description, image, enabled, price, unit)
- VALUES (%s, %s, %s, %s, %s, %s::boolean, %s, %s);
+ INSERT INTO offlineshop.items (shop, id, name, description, image, enabled, price, unit, fiat_base_multiplier)
+ VALUES (%s, %s, %s, %s, %s, %s::boolean, %s, %s, %s);
"""
items = res.fetchall()
insert_to_pg(q, items)
@@ -703,6 +704,19 @@ def migrate_ext(sqlite_db_file, schema, ignore_missing=True):
VALUES (%s, %s, %s, %s, %s, %s);
"""
insert_to_pg(q, res.fetchall())
+ elif schema == "scrub":
+ # SCRUB LINKS
+ res = sq.execute("SELECT * FROM scrub_links;")
+ q = f"""
+ INSERT INTO scrub.scrub_links (
+ id,
+ wallet,
+ description,
+ payoraddress
+ )
+ VALUES (%s, %s, %s, %s);
+ """
+ insert_to_pg(q, res.fetchall())
else:
print(f"❌ Not implemented: {schema}")
sq.close()